Matrix Subtraction Calculator
Matrix A
Matrix B
Result
A Matrix Subtraction Calculator is a tool that performs subtraction on two matrices. It takes as input two matrices of the same size and calculates the resulting matrix after subtracting the corresponding elements. Here's what you need to know about a Matrix Subtraction Calculator:
- Input: The calculator should have input fields to allow users to enter the dimensions (rows and columns) of the matrices and the values of each element in the matrices.
- Validation: The input values should be validated to ensure that they are numbers and that both matrices have the same dimensions for subtraction.
- Matrix Representation: Matrices are typically represented in a tabular form, where rows and columns display the matrix elements. A 2x2 matrix, for example, will have four input fields for the four elements.
- Subtraction Process: The calculator should perform the subtraction by subtracting the corresponding elements of both matrices. For example, the element at position (i, j) in the result matrix will be the subtraction of the elements at position (i, j) in the two input matrices.
- Displaying the Result: The resulting matrix should be displayed on the calculator after the subtraction is performed.
- Example: For example, if the user inputs two 2x2 matrices: Matrix A:Copy code
3 5 2 7
Matrix B:Copy code1 4 6 3
The resulting matrix (Matrix A - Matrix B) will be:diffCopy code2 1 -4 4
- Error Handling: The calculator should handle errors gracefully, such as invalid input or mismatched dimensions of the input matrices.
FAQs
How do you do matrix subtraction?
Matrix subtraction involves subtracting corresponding elements of two matrices of the same size. For example, to subtract Matrix A from Matrix B, you subtract each element of Matrix A from the corresponding element of Matrix B.
What is a matrix subtraction?
Matrix subtraction is an operation performed on two matrices of the same size, resulting in a new matrix with the same dimensions. It is used to find the difference between two matrices.
Can you subtract a 3x2 and a 2x3 matrix?
No, you cannot directly subtract a 3x2 matrix from a 2x3 matrix because their dimensions are different. Matrix subtraction is only possible when both matrices have the same number of rows and columns.
How do you subtract two by two matrices?
To subtract two 2x2 matrices, subtract the corresponding elements in each matrix. For example, to subtract Matrix A from Matrix B:
cssCopy codeA = [a b]
[c d]
B = [e f]
[g h]
Result (A - B) = [(a - e) (b - f)]
[(c - g) (d - h)]
What is the rule for matrix operations?
Matrix operations (addition, subtraction, and multiplication) are performed element-wise, meaning corresponding elements of the matrices are combined according to the operation being applied.
How do you solve a matrix equation?
To solve a matrix equation Ax = B, where A is a matrix, x is a column vector of unknowns, and B is a column vector, you need to find the inverse of matrix A (if it exists) and then multiply both sides of the equation by the inverse of A.
How do you subtract columns in a matrix?
To subtract two columns in a matrix, simply subtract the corresponding elements of the two columns. For example, to subtract Column B from Column A:
lessCopy codeA = [a b c]
[d e f]
[g h i]
Subtracting Column B from Column A: (A - B)
Result = [a - d b - e c - f]
[d - e e - f f - h]
[g - j h - k i - l]
What is the program for subtraction of two matrix?
A program for matrix subtraction can be written in any programming language. Here's a simple example in Python:
pythonCopy codedef subtract_matrices(matrix_a, matrix_b):
result_matrix = []
for i in range(len(matrix_a)):
row = []
for j in range(len(matrix_a[0])):
row.append(matrix_a[i][j] - matrix_b[i][j])
result_matrix.append(row)
return result_matrix
# Example usage:
matrix_a = [[1, 2], [3, 4]]
matrix_b = [[5, 6], [7, 8]]
result = subtract_matrices(matrix_a, matrix_b)
print(result) # Output: [[-4, -4], [-4, -4]]
What is the inverse of a 2x2 matrix?
The inverse of a 2x2 matrix is given by the formula:
scssCopy code[ a b ]
[ c d ]
Inverse = (1 / determinant) * [ d -b ]
[ -c a ]
Where the determinant of the 2x2 matrix is (ad - bc). Note that the inverse only exists if the determinant is not equal to zero.
Can you add a 2x2 and a 2x3 matrix?
No, you cannot directly add a 2x2 matrix to a 2x3 matrix because their dimensions are different. Matrix addition is only possible when both matrices have the same number of rows and columns.
How do you solve a 3x3 and 3x2 matrix?
To solve a 3x3 matrix equation (Ax = B) with a 3x2 matrix B, you need to find the inverse of matrix A (if it exists) and then multiply both sides of the equation by the inverse of A.
Can you subtract a matrix from both sides of an equation?
Yes, you can subtract a matrix from both sides of an equation as long as the matrix dimensions are compatible for subtraction.
Can you subtract matrix rows?
Yes, you can subtract matrix rows from each other. To subtract Row i from Row j, simply subtract the corresponding elements of Row i and Row j.
What are the rules for adding, subtracting, and multiplying matrices?
For matrix addition and subtraction, the matrices must have the same dimensions. For matrix multiplication, the number of columns in the first matrix must be equal to the number of rows in the second matrix.
How do you subtract matrices 2x3?
To subtract two 2x3 matrices, subtract the corresponding elements in each matrix. For example, to subtract Matrix A from Matrix B:
cssCopy codeA = [a b c]
[d e f]
B = [g h i]
[j k l]
Result (A - B) = [(a - g) (b - h) (c - i)]
[(d - j) (e - k) (f - l)]
How do you solve matrices 3x2 and 2x2?
To multiply a 3x2 matrix by a 2x2 matrix, the number of columns in the first matrix (3) must be equal to the number of rows in the second matrix (2). The resulting matrix will be a 3x2 matrix.
How do you subtract matrices with variables?
To subtract matrices with variables, simply subtract the corresponding elements that contain the variables.
What are the four basic operations used in matrices?
The four basic operations used in matrices are addition, subtraction, multiplication, and division.
What is the rule for inverse matrix?
The rule for finding the inverse of a matrix is that the matrix must be square (i.e., the number of rows is equal to the number of columns), and its determinant must be non-zero for the inverse to exist.
How do you find the inverse of a matrix?
To find the inverse of a matrix, you can use various methods such as Gaussian elimination, adjugate matrix method, or using the formula for the inverse of a 2x2 matrix.
How do you solve a matrix quickly?
The process of solving a matrix equation quickly involves using efficient matrix manipulation techniques like Gaussian elimination, LU decomposition, or matrix factorization methods.
What is the basic matrix formula?
The basic matrix formula includes operations like addition, subtraction, and multiplication of matrices.
How do you subtract rows and columns?
To subtract rows or columns, simply subtract the corresponding elements of the rows or columns.
How do you subtract two columns from each other?
To subtract two columns from each other, subtract the corresponding elements of the two columns.
How do you subtract two functions from each other?
To subtract two functions, subtract their corresponding output values at each point.
How do I subtract one column from another in numbers?
To subtract one column from another in numbers (a spreadsheet software), simply use the subtraction formula "=Column1 - Column2".
Can you subtract from both sides of an equation?
Yes, you can subtract the same value from both sides of an equation without changing the equality.
Which formula can you use to subtract the 2 quantities?
To subtract two quantities, simply use the subtraction formula: Result = Quantity1 - Quantity2.
What is the rule for adding and subtracting functions?
To add or subtract functions, combine or subtract their corresponding terms.
Can you subtract a 2x2 and a 3x2 matrix?
No, you cannot directly subtract a 2x2 matrix from a 3x2 matrix because their dimensions are different. Matrix subtraction is only possible when both matrices have the same number of rows and columns.
Can you subtract a 2x3 and 3x2 matrix?
No, you cannot directly subtract a 2x3 matrix from a 3x2 matrix because their dimensions are different. Matrix subtraction is only possible when both matrices have the same number of rows and columns.
What are the properties of matrix subtraction?
Matrix subtraction is commutative, associative, and has an identity element (the matrix with all elements as zeros).
How do you solve a 2x3 and 3x2 matrix?
To solve a 2x3 matrix equation (Ax = B) with a 3x2 matrix B, you need to find the inverse of matrix A (if it exists) and then multiply both sides of the equation by the inverse of A.
How do you solve a 2x3 matrix equation?
To solve a 2x3 matrix equation (Ax = B), where A is a 2x3 matrix and B is a column vector, you need to find the inverse of matrix A (if it exists) and then multiply both sides of the equation by the inverse of A.
How do you solve a 2x2 and 2x1 matrix?
To solve a 2x2 matrix equation (Ax = B) with a 2x1 matrix B, you need to find the inverse of matrix A (if it exists) and then multiply both sides of the equation by the inverse of A.
What is the subtraction of two matrices?
The subtraction of two matrices involves subtracting corresponding elements of the matrices to obtain a new matrix with the same dimensions.
What is the rule in subtracting variables?
When subtracting variables, combine like terms (variables with the same exponent).
Can you add a 2x2 and a 2x3 matrix?
No, you cannot directly add a 2x2 matrix to a 2x3 matrix because their dimensions are different. Matrix addition is only possible when both matrices have the same number of rows and columns.
Is matrix subtraction commutative?
No, matrix subtraction is not commutative. The order of matrices matters in subtraction. For example, if you subtract Matrix A from Matrix B, the result will be different from subtracting Matrix B from Matrix A.
What are the 8 types of matrices?
The 8 types of matrices include square matrices, rectangular matrices, zero matrices, identity matrices, diagonal matrices, scalar matrices, symmetric matrices, and skew-symmetric matrices.
What makes a matrix not invertible?
A matrix is not invertible (non-invertible or singular) if its determinant is equal to zero. In this case, the matrix does not have an inverse.
Why do we find the inverse of a matrix?
Finding the inverse of a matrix is useful for solving systems of linear equations, finding the division of matrices, and solving other matrix-related problems.
What is an inverse in a 3x3 matrix?
The inverse of a 3x3 matrix is a matrix that, when multiplied with the original matrix, results in the identity matrix.
How do you solve a 3x3 matrix problem?
To solve a 3x3 matrix problem, you may use various techniques like Gaussian elimination, matrix factorization methods, or using online calculators.
What is matrix problem-solving technique?
Matrix problem-solving techniques involve using matrix operations and methods to solve problems related to systems of linear equations, transformations, and other mathematical applications.
What is the first step to solve a matrix?
The first step to solve a matrix problem is to determine the type of matrix operation required (addition, subtraction, multiplication, etc.) and ensure the matrices have compatible dimensions for the operation.
How hard is matrix algebra?
Matrix algebra can be challenging, especially when dealing with large matrices or complex operations. However, with practice and understanding of the rules, it becomes manageable.
How to do matrix step by step?
To perform matrix operations step by step, follow these general steps: Write down the matrices, apply the desired operation element-wise, and simplify the resulting matrix.
What is the formula for solving a matrix?
The formula for solving a matrix equation (Ax = B) involves finding the inverse of matrix A (if it exists) and then multiplying both sides of the equation by the inverse of A to isolate x.
What is a matrix in math for dummies?
A matrix is a two-dimensional array of numbers or variables arranged in rows and columns used in various mathematical and computational applications.
How do you subtract two columns from each other?
To subtract two columns from each other, subtract the corresponding elements of the two columns.
How do you subtract multiple columns?
To subtract multiple columns from each other, subtract the corresponding elements of the columns.
How does column subtraction work?
Column subtraction works by subtracting corresponding elements of two or more columns in a matrix.
How do you add and subtract columns?
To add or subtract columns, simply add or subtract the corresponding elements of the columns.
How do you subtract two functions from each other?
To subtract two functions, subtract their corresponding output values at each point.
How do I subtract one column from another in numbers?
To subtract one column from another in numbers (a spreadsheet software), simply use the subtraction formula "=Column1 - Column2."
Can you subtract from both sides of an equation?
Yes, you can subtract the same value from both sides of an equation without changing the equality.
Which formula can you use to subtract the 2 quantities?
To subtract two quantities, simply use the subtraction formula: Result = Quantity1 - Quantity2.
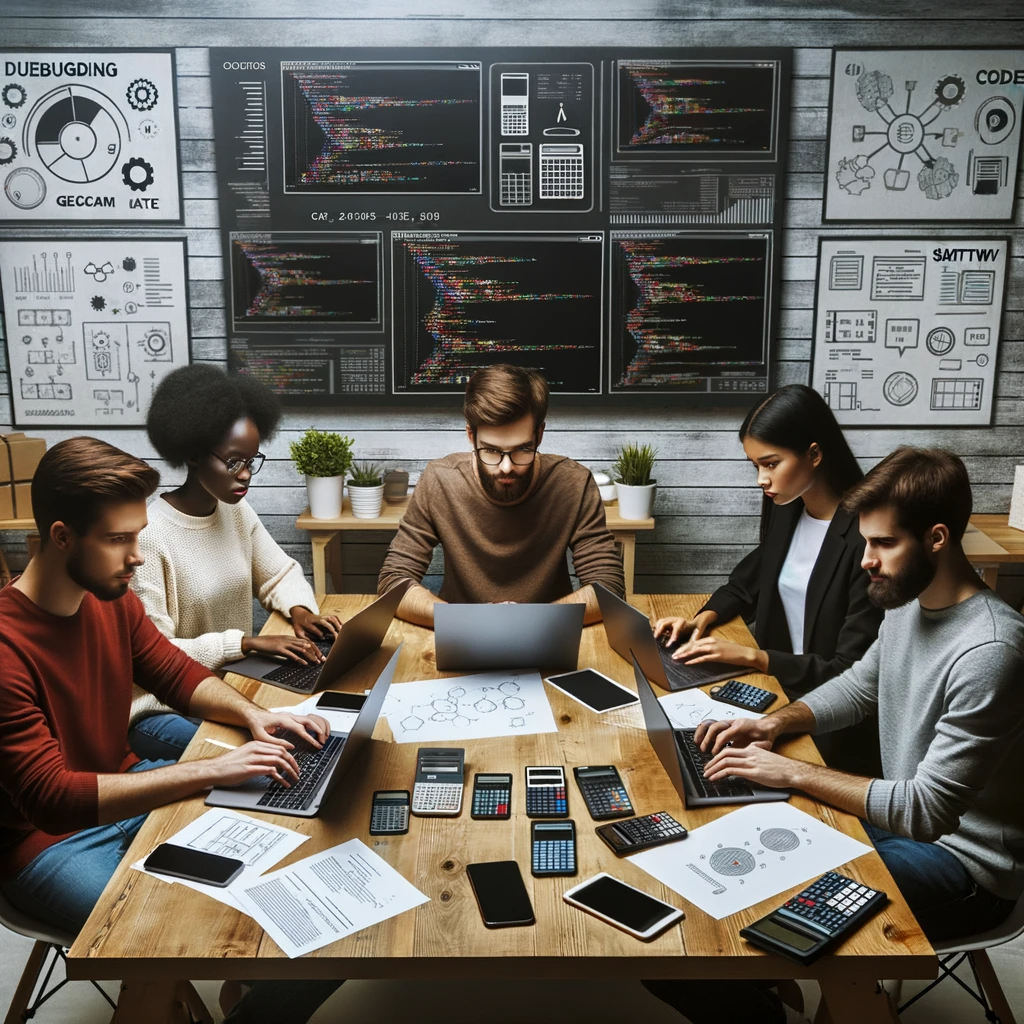
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.