To calculate someone’s age, we first need their date of birth. In this guide, we will learn how to easily obtain user input for birthdate in Python and convert it into a usable format for age calculations.
Next, we will dive into the logic for age calculation in Python. You will learn how to implement the necessary calculations to accurately determine someone’s age based on their birthdate.
Python is known for its rich library ecosystem, and there are libraries that can simplify the age calculation process even further. We will explore some popular libraries and demonstrate how to utilize them for age calculation in Python.
In conclusion, this guide will equip you with the knowledge and skills to effortlessly calculate someone’s age using Python. By the end of this article, you will have a deep understanding of age calculation techniques and be ready to implement them in your own projects.
Key Takeaways:
- Python offers powerful tools for age calculation based on date and time manipulation.
- You can obtain user input for birthdate in Python using simple techniques.
- Implementing the logic for age calculation requires understanding basic arithmetic operations.
- There are libraries in Python that can simplify the age calculation process.
- By mastering age calculation in Python, you can enhance various applications and provide personalized experiences.
Understanding Date and Time in Python
Before we begin calculating age, it is essential to have a basic understanding of how Python handles date and time. Python provides a robust set of tools and libraries for working with date and time objects, allowing us to perform various operations like date parsing, formatting, arithmetic, and more. Let’s dive into the fundamentals of date and time manipulation in Python.
Python’s standard library includes the datetime module, which offers classes and methods to work with dates, times, and time intervals. With this module, you can represent dates and times as objects, enabling precise and accurate calculations.
One of the key classes in the datetime module is the datetime class, which combines both date and time information. It allows us to create datetime objects that represent specific points in time, such as birthdays, appointments, or any other significant event.
Here is an example that demonstrates the creation of a datetime object:
<datetime.datetime(2022, 12, 31, 23, 59, 59)>
In the above example, we have a datetime object representing December 31, 2022, at 11:59:59 PM.
Python also provides the date and time classes, which represent dates and times separately. These classes are useful when we only need to work with either dates or times, rather than both simultaneously.
Furthermore, Python allows us to perform various operations on date and time objects. We can perform arithmetic operations such as addition, subtraction, and comparison. This flexibility enables us to calculate time intervals, find the difference between two dates, and compare dates to determine their order.
Working with Date and Time Objects
Let’s explore some common operations we can perform with date and time objects in Python:
- Creating date, time, and datetime objects
- Accessing individual components of date and time objects (year, month, day, hour, minute, second)
- Formatting dates and times as strings
- Extracting specific information from datetime objects (e.g., weekday, time zone)
- Performing arithmetic operations on date and time objects
- Comparing dates and times
Now that we have a solid understanding of working with date and time objects in Python, we are ready to dive into calculating someone’s age. Let’s move on to the next section and learn how to obtain user input for birthdate.
Obtaining User Input for Birthdate
In order to calculate someone’s age, we first need to obtain their date of birth as input. This can be achieved in Python through a variety of methods. Let’s explore two common approaches:
1. Using the input() function
The input() function allows us to prompt the user for input and retrieve their response as a string. To obtain the birthdate, we can use a simple prompt like:
Enter your birthdate (YYYY-MM-DD):
The user can then input their birthdate in the specified format. We can store this input in a variable for further processing and age calculation.
“`python
birthdate = input(“Enter your birthdate (YYYY-MM-DD): “)
“`
2. Utilizing specialized Python libraries
Python offers powerful libraries that streamline the process of obtaining user input for birthdates. One such library is the datetime
library, which provides classes and methods for manipulating dates and times.
Here’s an example of how we can use the datetime
library to obtain the birthdate:
“`python
from datetime import datetime
birthdate = input(“Enter your birthdate (YYYY-MM-DD): “)
birthdate = datetime.strptime(birthdate, “%Y-%m-%d”)
“`
In this example, we use the strptime()
method from the datetime
library to convert the user’s input into a datetime
object. This allows for easier manipulation and calculation of age.
By obtaining the user’s birthdate, we can proceed to calculate their age using the techniques covered in the subsequent sections.
Age Calculation Logic
Now that we have the birthdate, we can proceed to calculate the age. In this section, we will guide you through the process of implementing the logic for age calculation in Python.
Calculating someone’s age in Python involves a simple formula that takes the current date and the birthdate into account. By subtracting the birthdate from the current date, we can determine the number of days, months, and years that have passed since the person’s birth.
Here’s the age calculation formula in Python:
age = current_date – birthdate
Once you have the age, you can further refine the result by extracting specific details like the number of years, months, and days.
To ensure accurate age calculation, consider the following factors:
- Leap years: Take into account leap years when calculating the age.
- Birthdate validation: Validate the user input to ensure it follows the expected date format and falls within a reasonable range.
- Current date: Obtain the current date from the system to calculate the age accurately.
Implementing the age calculation logic in Python may vary depending on your specific use case and the libraries you choose to utilize. In the next section, we will explore some libraries that can simplify the age calculation process even further.
Age Calculation Using Libraries
Python provides a range of libraries that can simplify age calculation processes. These libraries offer ready-to-use functions and methods, effectively reducing the complexity of coding age calculation logic from scratch. Let’s explore some of the popular libraries and see how they can be utilized for age calculation in Python.
1. datetime Library
The datetime library in Python allows you to work with dates, times, and intervals. It provides the date
class, which can be used to create date objects that store individual components like year, month, and day. By leveraging this library, you can effortlessly calculate the age of a person based on their birthdate.
2. dateutil.relativedelta Library
The dateutil.relativedelta library is a powerful extension of the datetime library. It allows you to perform complex date calculations, such as determining the difference between two dates in terms of years, months, days, hours, minutes, and seconds. With this library, you can accurately calculate someone’s age by considering different factors, such as leap years and varying month lengths.
3. arrow Library
The arrow library provides a simple and intuitive interface for working with dates and times in Python. It offers a wide range of functionalities, including date manipulation, formatting, parsing, and time zone conversion. Using arrow, you can easily calculate a person’s age by subtracting their birthdate from the current date and extracting the relevant information.
4. age * Library
The age library is specifically designed for age calculation in Python. It provides a straightforward and concise syntax for calculating someone’s age based on their birthdate. With this library, you can easily determine the age in years, months, and days, all in a single line of code.
Keep in mind that before using any library, you need to ensure it is installed on your system. To install a library in Python, you can use package managers such as
pip
orconda
. For example, runningpip install datetime
will install the datetime library.
By leveraging these libraries, age calculation in Python becomes more efficient and less error-prone. Depending on your specific needs and preferences, you can choose the library that best suits your requirements and seamlessly integrate it into your code.
Conclusion
Summarizing age calculation in Python, we have explored various techniques and libraries to effortlessly determine someone’s age. By understanding the fundamentals of date and time manipulation in Python, obtaining user input for birthdate, and implementing the age calculation logic, we can now perform accurate age calculations.
Python provides several libraries that make age calculation even more straightforward. Libraries like datetime, dateutil, and arrow offer functionalities to handle dates, calculate time differences, and extract specific components from a date object. These libraries save time and effort by providing ready-to-use functions and simplifying the age calculation process.
It is essential to remember that age calculation in Python relies on accurate and valid data input. It is crucial to handle user input validation to ensure the birthdate is in the correct format and falls within reasonable ranges. Additionally, considering factors like time zones and leap years can enhance the accuracy of age calculations.
In conclusion, age calculation in Python opens up a world of possibilities for applications like age verification, demographic analysis, and targeted marketing campaigns. With the knowledge gained from this article, you can confidently perform age calculations and incorporate them into your Python projects.
FAQ
How do I calculate someone’s age in Python?
To calculate someone’s age in Python, you can use various techniques. You can subtract the person’s birth year from the current year or utilize Python’s built-in datetime module to calculate the difference between two dates.
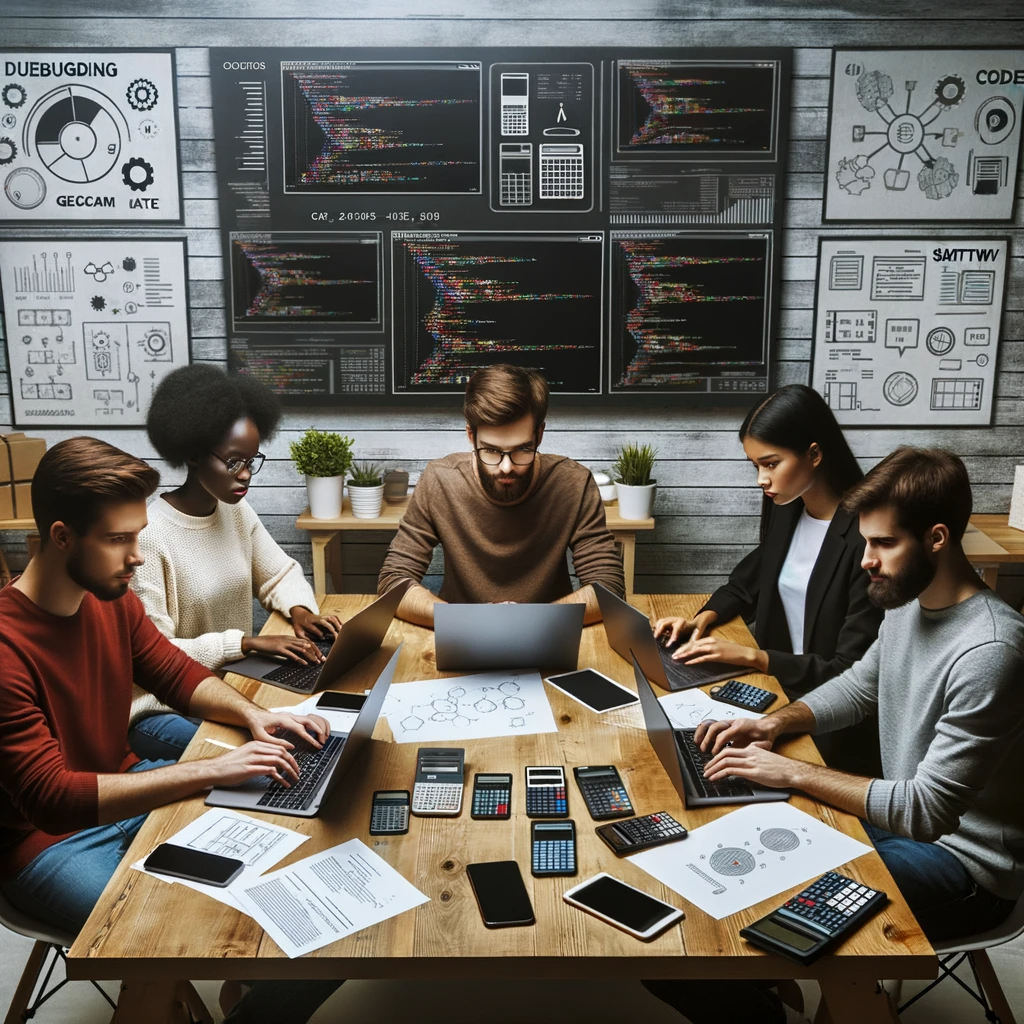
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.