**1. Understanding the Role of Threading in Software Development**
Threading plays a crucial role in software development by enabling concurrent execution of tasks. In simple terms, threading refers to the technique of dividing a program into multiple threads, allowing them to run simultaneously. Each thread represents an independent pathway of execution, capable of performing its own set of tasks.
The primary purpose of threading is to maximize the utilization of available system resources, such as CPU and memory. By utilizing multiple threads, developers can achieve parallelism and increase the overall efficiency and responsiveness of their software. Threading also facilitates better utilization of multi-core processors, which are now prevalent in modern computing devices. Consequently, developers can leverage threading to design applications that can handle complex tasks, process large datasets, and deliver a smooth user experience.
**2. Benefits of Using Threading in Software Development**
Parallel processing and concurrency have become essential aspects of modern software development. Threading, a technique that involves dividing a program into multiple threads, offers numerous benefits in this regard.
One of the primary advantages of using threading in software development is improved performance and responsiveness. By utilizing multiple threads, developers can effectively utilize the available resources of a system, leading to faster execution of tasks. For instance, in a multi-threaded application, one thread can be responsible for handling user interface updates, while another thread performs data processing in the background. This allows the application to remain responsive to user interactions, even when time-consuming operations are being performed.
Furthermore, threading enables better resource management and utilization. By dividing a program into smaller threads, developers can allocate system resources more efficiently. This results in enhanced scalability, allowing the software to handle larger workloads and utilize available processor cores to their fullest potential. Additionally, threading facilitates the development of more complex and intricate applications, as different threads can be dedicated to distinct functionalities, reducing the chance of conflicts or unwanted dependencies.
In summary, threading provides substantial benefits in terms of performance, responsiveness, resource management, and scalability. By judiciously implementing threading techniques in software development, developers can take advantage of parallel processing and enable their applications to perform optimally in a multi-core, concurrent system environment.
**3. Considerations for Applying Threading in Software Development**
When considering the application of threading in software development, there are several important factors to keep in mind. First and foremost, it is crucial to understand the nature of the problem you are trying to solve and whether threading is the most suitable solution. Threading can be beneficial in scenarios where there are multiple tasks that can be executed concurrently or when there is a need for parallel processing. However, it is essential to evaluate whether the potential gains in performance and efficiency outweigh the complexity and challenges that threading can introduce.
Another consideration is the design and structure of your codebase. Threading can introduce concurrency issues such as race conditions and deadlocks, which can be difficult to debug and resolve. Therefore, it is crucial to have a solid understanding of threading concepts and best practices to ensure that your code is thread-safe and resilient. Additionally, incorporating threading into an existing codebase may require significant modifications and can potentially introduce compatibility issues. Therefore, thoroughly evaluating the impact of threading on your codebase and considering the long-term maintenance and scalability aspects is vital before applying threading in software development.
**4. Evaluating the Need for Threading in Your Code**
When evaluating the need for threading in your code, it is important to consider the specific requirements and characteristics of your application. Threading can be beneficial in situations where you need to perform multiple tasks concurrently or improve the responsiveness of your application.
One aspect to consider is whether your application is computationally intensive and can benefit from parallel processing. Threading allows you to divide the workload among multiple threads, leveraging the processing power of multi-core processors. This can significantly improve the performance of your application, especially when dealing with large datasets or complex calculations. Additionally, threading can be useful in scenarios where your application needs to handle multiple external requests simultaneously, such as when developing server applications or handling network communications. By allowing different threads to handle different requests concurrently, you can enhance the responsiveness and scalability of your application.
**5. Common Use Cases for Threading in Software Development**
Threading in software development offers a range of common use cases that can enhance the performance and functionality of an application. One common use case is in graphical user interfaces (GUIs), where threading can be used to keep the user interface responsive while performing heavy computations or network operations in the background. By separating these tasks into different threads, the application can provide a seamless user experience without freezing or becoming unresponsive.
Another common use case for threading is in server applications, especially those that need to handle multiple client connections simultaneously. By assigning each incoming client connection to its own thread, the server can handle multiple requests concurrently and maximize the overall throughput. This can greatly enhance the scalability and responsiveness of the server, allowing it to efficiently process a large number of client requests in parallel. Threading enables server applications to make the most of the available system resources and provide a smoother experience for the clients.
**6. Potential Challenges and Pitfalls of Threading in Software Development**
Threading in software development, while beneficial in many cases, can also present a set of potential challenges and pitfalls for developers to navigate. One primary challenge is the issue of concurrency and race conditions. When multiple threads access shared resources simultaneously, conflicts can arise, leading to unexpected behavior and difficult-to-debug issues. It requires careful synchronization techniques, such as locks and semaphores, to ensure that data integrity is maintained and prevent race conditions from occurring.
Another challenge is the complexity introduced by threading. As the number of threads increases, the code becomes harder to understand and reason about. Debugging threaded code can be particularly challenging, as timing-dependent bugs can be difficult to reproduce consistently. Additionally, the introduction of shared resources and the need for synchronization can lead to increased code complexity and potential for errors. It is essential for developers to thoroughly understand the impact of threading on their codebase and be diligent in implementing proper design patterns and synchronization mechanisms to mitigate these challenges.
Overall, while threading can offer significant benefits in terms of performance and responsiveness, the potential challenges and pitfalls should not be overlooked. Careful planning, thorough understanding of threading concepts, and adherence to best practices can help developers overcome these difficulties and effectively leverage threading in software development.
**7. Alternatives to Threading in Software Development**
There are instances in software development where the use of threading may not be the most suitable approach. In such cases, alternative methods can be employed to achieve desired outcomes. One alternative to threading is the use of asynchronous programming. By leveraging concepts like callbacks, promises, and async-await, developers can design code that runs concurrently without the need for multiple threads. Asynchronous programming offers a more scalable and efficient solution, especially when dealing with I/O-bound operations such as network requests and file operations.
Another alternative to threading is event-driven programming. This paradigm revolves around the concept of triggering actions based on specific events or signals. Instead of relying on threads to handle concurrent tasks, event-driven programming utilizes event handlers to respond to events as they occur. This approach can be particularly useful in scenarios where responsiveness and real-time processing are crucial, such as user interfaces and game development. By decoupling tasks into independent event-driven modules, developers can achieve efficient and scalable code execution without the complexities and overhead associated with threading.
• Asynchronous programming: By leveraging concepts like callbacks, promises, and async-await, developers can design code that runs concurrently without the need for multiple threads.
• Event-driven programming: This paradigm revolves around triggering actions based on specific events or signals.
• Instead of relying on threads to handle concurrent tasks, event-driven programming utilizes event handlers to respond to events as they occur.
• This approach is particularly useful in scenarios where responsiveness and real-time processing are crucial, such as user interfaces and game development.
• Decoupling tasks into independent event-driven modules allows for efficient and scalable code execution without the complexities and overhead associated with threading.
**8. Best Practices for Implementing Threading in Your Code**
When implementing threading in your code, it is crucial to follow best practices to ensure efficient and reliable performance. Firstly, it is important to properly manage the number of threads your application uses. Creating an excessive number of threads can lead to resource contention and increased overhead. On the other hand, having too few threads may result in underutilization of available resources. It is recommended to analyze the workload and design your thread pool accordingly, considering factors such as the number of processors, the nature of the tasks, and the overall system performance.
In addition, proper synchronization mechanisms should be employed to avoid race conditions and ensure thread safety. Synchronization primitives such as locks, mutexes, and semaphores can be used to control access to shared resources. However, it is essential to use these mechanisms judiciously and avoid excessive locking, as it can lead to contention and degrade performance. As an alternative, consider using higher-level synchronization constructs like concurrent data structures or higher-level abstractions provided by your programming language or framework.
These best practices will help you harness the power of threading in your code while avoiding potential pitfalls and ensuring the reliability and performance of your application. However, it is important to note that the specific considerations and approaches may vary depending on your programming language, platform, and the unique requirements of your software project. Therefore, it is recommended to continuously evaluate and adapt these best practices to optimize the threading implementation in your code.
What is the role of threading in software development?
Threading allows for concurrent execution of tasks, improving the performance and responsiveness of software applications.
What are the benefits of using threading in software development?
Threading can enhance parallelism, improve resource utilization, increase responsiveness, and enable efficient multitasking in software development.
What considerations should be kept in mind when applying threading in software development?
Developers should consider factors such as shared resources, synchronization, potential race conditions, and the complexity of managing concurrent threads.
How can one evaluate the need for threading in their code?
The need for threading depends on the nature of the tasks involved, the potential for parallel execution, and the performance requirements of the software application.
What are some common use cases for threading in software development?
Threading is commonly used in scenarios such as handling user interface responsiveness, performing background tasks, executing multiple I/O operations simultaneously, and implementing parallel algorithms.
What challenges and pitfalls should developers be aware of when using threading in software development?
Developers should be mindful of issues like thread synchronization, deadlock, livelock, and the potential for performance degradation due to excessive context switching.
Are there any alternatives to threading in software development?
Yes, alternatives include using asynchronous programming models, event-driven architectures, or leveraging parallel processing frameworks, depending on the specific requirements and constraints of the application.
What are some best practices for implementing threading in code?
Some best practices include minimizing shared state, properly synchronizing access to shared resources, using thread-safe data structures, avoiding unnecessary thread creation, and thoroughly testing the code for thread safety.
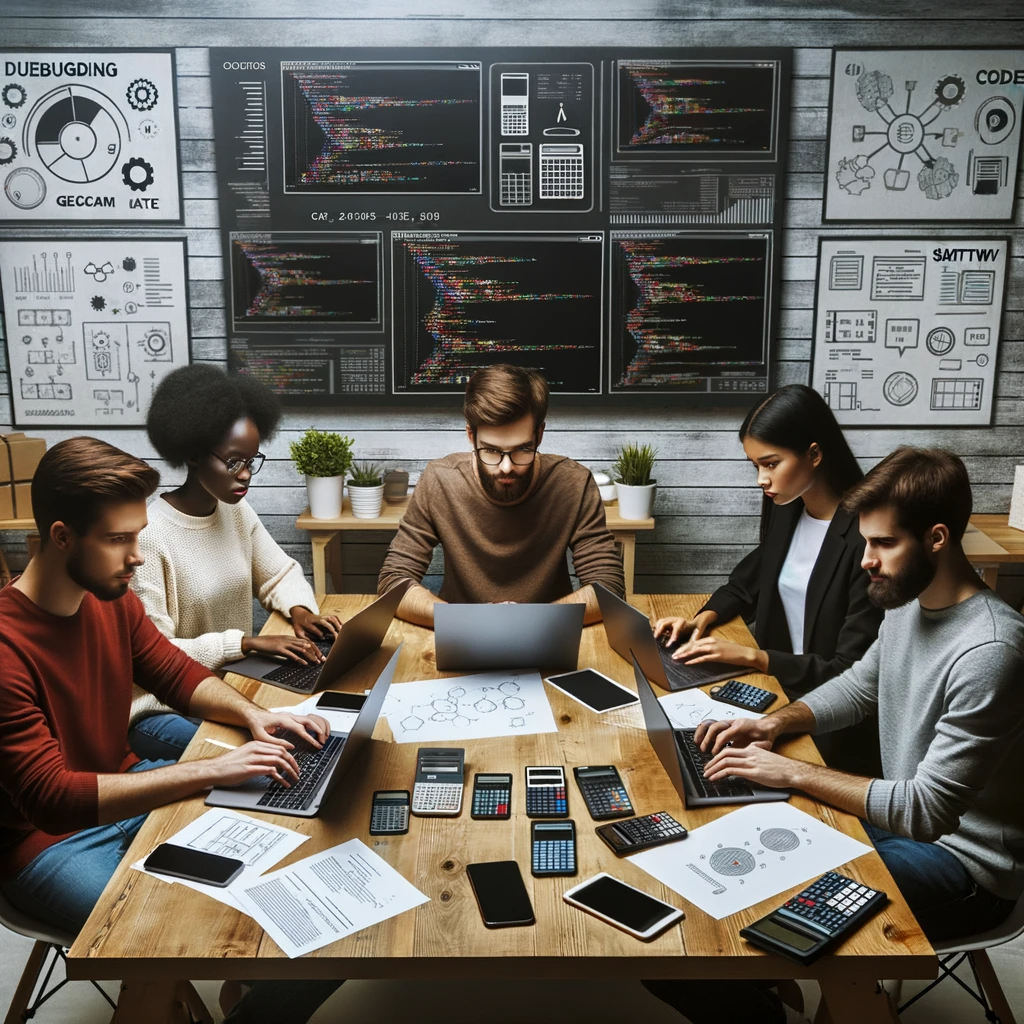
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.