A Left-Leaning Red-Black Tree (LLRB) is a self-balancing binary search tree where each node has a color (red or black). LLRBs are a simplified variant of red-black trees and maintain balance through color constraints and left-leaning properties. They provide efficient insertion, deletion, and search operations, making them suitable for various applications requiring ordered data storage.
Left-Leaning Red-Black Tree Calculator
Table Name: LLRBTree
Field Name | Data Type | Description |
---|---|---|
NodeID | Integer or UniqueID | Unique identifier for each tree node. |
Key | Comparable Data Type | The value or key associated with the tree node. |
Value | Any Data Type | The data or value associated with the key. |
Color | Enum (Red/Black) | The color of the node (Red or Black). |
LeftChildID | Integer or UniqueID | Identifier of the left child node. |
RightChildID | Integer or UniqueID | Identifier of the right child node. |
ParentID | Integer or UniqueID | Identifier of the parent node. |
IsRoot | Boolean | Indicates whether the node is the root of the tree. |
IsLeaf | Boolean | Indicates whether the node is a leaf (NIL) node. |
IsNull | Boolean | Indicates whether the node is NULL or empty. |
In the LLRB tree table structure described above:
NodeID
is a unique identifier for each node in the tree.Key
represents the value or key associated with the node.Value
stores the data associated with the key.Color
specifies whether the node is red or black.LeftChildID
andRightChildID
hold the identifiers of the left and right child nodes, respectively.ParentID
stores the identifier of the parent node.IsRoot
,IsLeaf
, andIsNull
are boolean fields that provide additional information about the node’s position in the tree.
You would use this table to implement an LLRB tree by performing database operations (insertions, deletions, and searches) based on the defined structure and constraints of an LLRB tree.
Remember that this table structure is a conceptual representation, and the actual implementation may vary depending on the programming language or database system you are using. Additionally, you would need to define methods or procedures to manipulate the LLRB tree using the data stored in this table.
FAQs
What is the maximum height of left-leaning red-black tree?
The maximum height of a left-leaning red-black tree is approximately 2*log2(n+1), where ‘n’ is the number of nodes in the tree. This height ensures that the tree remains balanced and satisfies the properties of a red-black tree.
What is left-leaning red-black tree algorithm?
A left-leaning red-black tree is a type of self-balancing binary search tree that is a variation of the standard red-black tree. It maintains the properties of a red-black tree but uses a simpler algorithm for insertion and deletion. In a left-leaning red-black tree, a red node can have a red left child but not a red right child. This makes the tree lean to the left, which simplifies the balancing process.
Can a red-black tree be unbalanced?
No, a properly implemented red-black tree should not be unbalanced. Red-black trees are designed to maintain balance, ensuring that the height of the tree remains logarithmic in relation to the number of nodes, which guarantees efficient search and insertion operations.
How do you check if a red-black tree is balanced?
A red-black tree is considered balanced if it satisfies the following properties:
- Every node is either red or black.
- The root node is black.
- Every leaf (NIL) node is black.
- Red nodes have only black children.
- For each node, any simple path from that node to any of its descendant leaf nodes contains the same number of black nodes.
If these properties are upheld, the red-black tree is balanced.
How do you measure the height of a leaning tree?
To measure the height of a leaning tree, you can use a clinometer or inclinometer. These devices allow you to measure the angle between your line of sight to the top of the tree and the ground. By using trigonometry and knowing the distance from your location to the base of the tree, you can calculate the tree’s height.
What limits the maximum height of a tree?
The maximum height of a tree is limited by factors such as the tree’s species, growth conditions, and genetic potential. In general, factors like gravity, structural stability, and the tree’s ability to transport water and nutrients to its uppermost branches limit its maximum height.
How do you turn a 2-4 tree into a red-black tree?
To convert a 2-4 tree into a red-black tree, you can follow these steps:
- Map 2-4 tree nodes to red-black tree nodes:
- 2-node in 2-4 tree becomes a black node in the red-black tree.
- 3-node in 2-4 tree becomes a black node with two red children in the red-black tree.
- 4-node in 2-4 tree becomes a black node with two red children in the red-black tree.
- Recolor the red-black tree to maintain red-black properties:
- Ensure that no two consecutive red nodes exist on any path from the root to a leaf.
- Adjust colors and rotations as needed to maintain balance.
This transformation ensures that the red-black tree retains its balancing properties while accommodating the nodes from the 2-4 tree.
What is red-black tree rule 4?
In red-black trees, rule 4 states that for every node, any simple path from this node to any of its descendant leaf nodes must contain the same number of black nodes. This property ensures that the tree remains balanced, with a logarithmic height relative to the number of nodes.
What is a 2-3-4 tree in data structure?
A 2-3-4 tree is a self-balancing tree data structure in which each node can have two, three, or four children. It is a type of B-tree and is used to maintain a sorted collection of data. The tree ensures that all leaf nodes are at the same level, and it can efficiently insert, delete, and search for data.
Can a 2-3 tree be unbalanced?
No, a properly implemented 2-3 tree should not be unbalanced. 2-3 trees are designed to maintain balance automatically during insertion and deletion operations to ensure that all leaf nodes remain at the same level, providing efficient search and retrieval.
What are the disadvantages of red-black tree?
Some disadvantages of red-black trees include:
- They may have slightly higher overhead than simpler data structures due to the color attributes and balancing operations.
- While red-black trees maintain balance well, they may not be as space-efficient as some other data structures.
- Insertion and deletion operations can be more complex than those in simpler data structures.
How do you check if a tree is perfectly balanced?
A tree is perfectly balanced if all its leaf nodes are at the same level. To check for perfect balance, traverse the tree from the root to all leaf nodes and ensure that the depth (level) of each leaf node is the same.
How do you balance an unbalanced tree?
Balancing an unbalanced tree typically involves restructuring the tree through rotations, node insertions, or deletions to ensure that it adheres to the balance criteria specific to its type (e.g., AVL tree, red-black tree). The exact procedure depends on the type of tree and the specific imbalance issue.
What does a balanced tree look like?
A balanced tree, such as an AVL tree or a red-black tree, has the following characteristics:
- All leaf nodes are at the same level.
- The height of the tree is logarithmic in relation to the number of nodes.
- The tree maintains its balance during insertions and deletions, ensuring efficient search operations.
What makes a tree balanced or unbalanced?
A tree is balanced when its structure ensures that all leaf nodes are at the same level, and its height is logarithmic in relation to the number of nodes. It becomes unbalanced when nodes are added or removed in a way that violates these balance criteria.
How do you tell which way a tree is leaning?
To determine which way a tree is leaning, observe the alignment of its branches and the trunk. The side with more weight, foliage, or growth typically indicates the direction of the lean. Additionally, you can use a plumb line or a clinometer to measure the angle of lean more accurately.
What is the very accurate tool to measure tree height?
One of the most accurate tools for measuring tree height is a clinometer or an inclinometer, often used with a rangefinder or a laser distance measurer. These tools allow you to measure the angle between your line of sight to the top of the tree and the ground, from which you can calculate the tree’s height using trigonometry.
What is the most accurate way to measure the height of a tree?
The most accurate way to measure the height of a tree is by using a clinometer or inclinometer in combination with a rangefinder or laser distance measurer. This method provides precise measurements by triangulating the angle and distance to the tree’s top.
Can you keep a tree at a certain height?
You can manage the height of certain tree species through pruning and trimming techniques. However, it’s essential to consult with an arborist or tree care professional to ensure proper and safe tree maintenance.
Is a tree with a mature height of 20 considered a large tree?
A tree with a mature height of 20 feet is generally considered a small to medium-sized tree. Large trees typically have mature heights well above 20 feet, often exceeding 50 feet or more, depending on the species.
What happens when a tree reaches maximum height?
When a tree reaches its maximum height, it typically continues to grow outward in terms of canopy size and girth. The tree may focus on producing branches and leaves rather than increasing its height significantly. Factors such as the species and environmental conditions play a role in determining a tree’s maximum height.
What is the formula for the height of a red-black tree?
The formula for the height of a red-black tree is approximately 2*log2(n+1), where ‘n’ is the number of nodes in the tree. This formula ensures that the red-black tree remains balanced and maintains its height within a logarithmic range.
What are the rules for red-black trees?
The rules for red-black trees include:
- Every node is either red or black.
- The root node is black.
- Every leaf (NIL) node is black.
- Red nodes have only black children.
- For each node, any simple path from that node to any of its descendant leaf nodes contains the same number of black nodes.
These rules ensure that the tree remains balanced and efficient for search and insertion operations.
How to plant 10 trees in 5 rows with 4 trees in each row?
To plant 10 trees in 5 rows with 4 trees in each row, you can arrange them as follows:
mathematicaCopy code
Row 1: X X X X Row 2: X X X X Row 3: X X Row 4: X X Row 5: X X
Each “X” represents a tree, and this arrangement fulfills the requirement of 10 trees in 5 rows with 4 trees in each row.
Why do people prefer red-black trees over?
It seems like your question is incomplete. If you provide more context or details, I’d be happy to answer your question about why people might prefer red-black trees over other data structures.
What is the red uncle rule in red-black trees?
The “red uncle” rule is not a standard term in red-black tree terminology. Red-black trees primarily follow the rules mentioned earlier. If you have a specific context or rule you’d like to inquire about, please provide more information.
Can a red-black tree have two red children?
In a red-black tree, a red node cannot have two red children. This rule is essential for maintaining the balance and properties of a red-black tree. Red nodes can only have black children to ensure that no two consecutive red nodes exist on any path from the root to a leaf.
Is a 2-4 tree the same as a 2-3-4 tree?
A 2-4 tree is closely related to a 2-3-4 tree, but they are not precisely the same. Both are self-balancing tree data structures, but in a 2-4 tree, nodes can have two, three, or four children. In a 2-3-4 tree, nodes can have two, three, or four data items and children, which makes it a more general representation.
What are the advantages of 2-3-4 tree?
Advantages of a 2-3-4 tree include:
- Self-balancing: It automatically maintains balance during insertions and deletions, ensuring efficient search operations.
- Supports a range of data items: Each node can have 2, 3, or 4 data items, making it versatile for various applications.
- Efficient for disk-based storage: 2-3-4 trees are used in database systems where data is stored on disk, thanks to their balanced nature.
What is the difference between B-tree and 2-4 tree?
The main difference between a B-tree and a 2-4 tree is in their node structure:
- In a B-tree, nodes can have a variable number of children, typically within a specified range. B-trees are used in databases and file systems.
- In a 2-4 tree, nodes can have two, three, or four children. 2-4 trees are a more specific type of self-balancing tree often used in memory-based data structures.
What is the disadvantage of 2-3 tree?
A disadvantage of a 2-3 tree is that it can have more complex node splitting and merging operations compared to other self-balancing trees, like AVL trees. This complexity can make implementations more challenging.
What is a perfectly unbalanced tree?
A perfectly unbalanced tree is not a standard term in tree data structures. Trees are typically classified as balanced or unbalanced based on their adherence to certain balance criteria. A perfectly unbalanced tree would likely be one that has no balance criteria and exhibits irregular and inefficient structure.
What is the maximum height of a 2-3 tree?
The maximum height of a 2-3 tree with ‘n’ nodes is approximately log3(n+1). This means that a 2-3 tree is relatively shallow and efficient for search operations, as its height remains logarithmic in relation to the number of nodes.
What are five properties of a red-black tree?
Five properties of a red-black tree include:
- Every node is either red or black.
- The root node is black.
- Every leaf (NIL) node is black.
- Red nodes have only black children.
- For each node, any simple path from that node to any of its descendant leaf nodes contains the same number of black nodes.
These properties ensure the tree’s balance and efficient operation.
Why is it called a red-black tree?
A red-black tree is named because each node in the tree is assigned a color, either red or black. This coloring scheme is essential for maintaining the tree’s balance and ensuring that it adheres to specific properties that guarantee efficient search and insertion operations.
What is the difference between AA tree and red-black tree?
AA trees and red-black trees are both self-balancing binary search trees, but they have different balancing mechanisms:
- Red-black trees use color attributes (red and black) and rotations to maintain balance.
- AA trees use a level-order traversal (also known as level-order splitting) and skewing to ensure balance.
AA trees are simpler to implement but may require more rotations than red-black trees for balancing.
Which tree is self-balancing?
Both red-black trees and AVL trees are examples of self-balancing trees. They automatically maintain balance during insertion and deletion operations, ensuring that the tree remains efficient for search and other operations.
How do you identify tree problems?
Identifying tree problems typically involves visual inspection and consideration of various factors, including:
- Visible signs of disease or decay in the trunk or branches.
- Leaning or unstable posture.
- Damaged or dead branches.
- Unusual growth patterns or symptoms such as wilting leaves.
- Pests or infestations.
- Soil and root health. For more accurate assessments, consulting an arborist or tree care professional is recommended.
Is any complete tree a balanced tree?
No, not every complete tree is necessarily a balanced tree. A complete tree is a tree in which all levels, except possibly the last one, are completely filled, reading from left to right. While complete trees can be balanced, they may not always be balanced depending on the specific tree structure and node values.
How do you make a self-balancing tree?
To make a self-balancing tree, you need to implement a balancing mechanism that automatically adjusts the tree’s structure during insertions and deletions to maintain balance. Common self-balancing trees include AVL trees, red-black trees, and splay trees. The choice of the tree structure depends on the specific requirements of your application.
How do you make a balanced tree?
To make a balanced tree, you can use self-balancing tree data structures like AVL trees or red-black trees. These data structures automatically maintain balance during insertions and deletions, ensuring that the tree’s height remains logarithmic in relation to the number of nodes.
Can a tree become unbalanced?
Yes, a tree can become unbalanced if nodes are inserted or deleted in a way that violates the tree’s balancing criteria. In self-balancing trees like AVL trees and red-black trees, balance is automatically restored through rotations or other operations when changes are made.
Are red-black trees always balanced?
Yes, properly implemented red-black trees are always balanced. They follow strict rules to ensure balance, such as the prohibition of two consecutive red nodes on any path from the root to a leaf. This balance property guarantees that the tree’s height remains logarithmic.
What is a full vs. balanced tree?
A full tree, also known as a complete tree, is a tree in which all levels, except possibly the last one, are completely filled, reading from left to right. A balanced tree, on the other hand, is a tree that maintains balance during insertions and deletions to ensure that its height remains logarithmic in relation to the number of nodes. While full trees are balanced, not all balanced trees are necessarily full.
Is an empty tree height balanced?
An empty tree is considered height balanced by default because it has no nodes, and therefore, its height is effectively zero. In height-balanced tree structures, balancing criteria primarily apply to non-empty trees.
How do you prune a lopsided tree?
Pruning a lopsided tree involves selectively removing branches and foliage to achieve a more balanced and aesthetically pleasing shape. It’s essential to consult with an arborist or tree care professional to ensure that pruning is done correctly and does not harm the tree.
How do you know if something is balanced or unbalanced?
Whether something is balanced or unbalanced depends on context. In the context of data structures like trees, balance is often assessed by specific criteria, such as maintaining a certain height relative to the number of nodes. In other contexts, balance may refer to the distribution of weight or resources, where balance implies an even distribution, while imbalance implies unevenness. To determine balance or imbalance, you need to consider the relevant criteria and context.
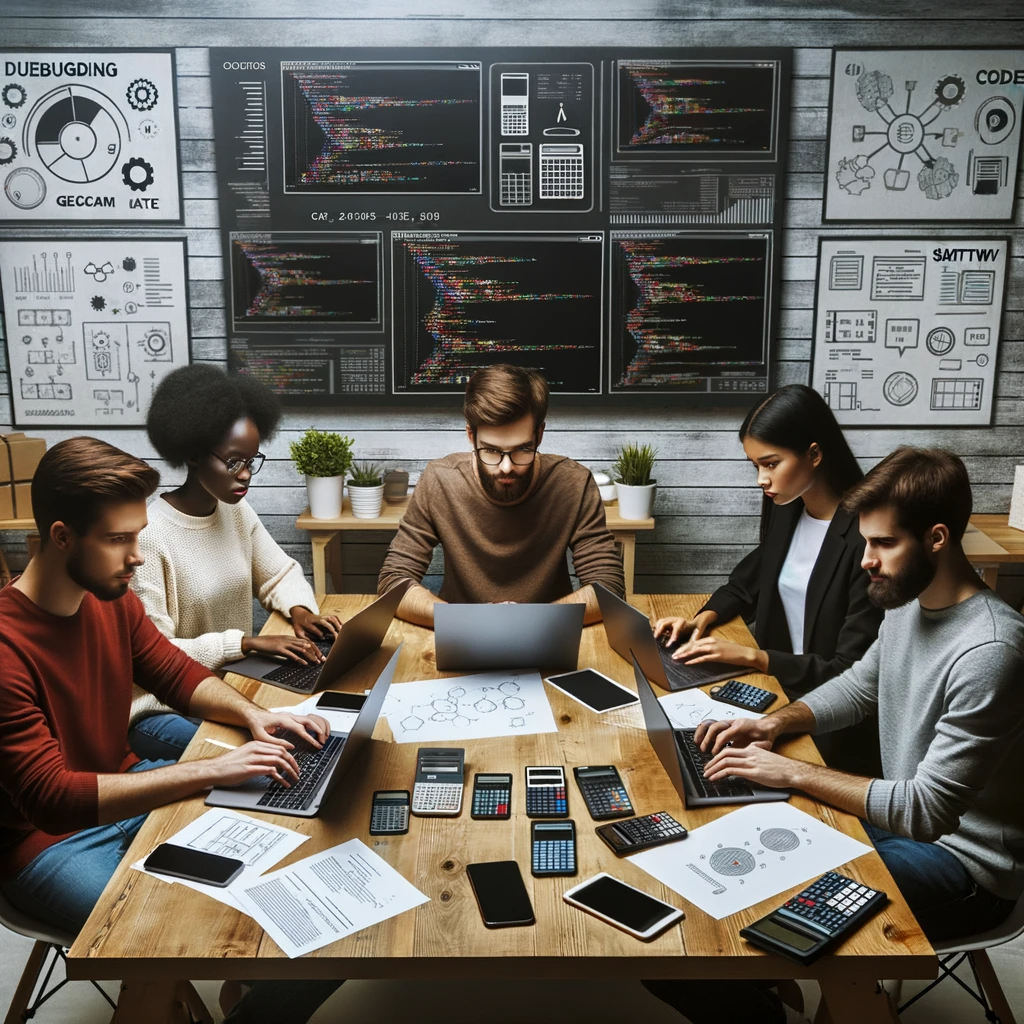
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.