In this article, we will explore the concept of time complexity in depth and provide step-by-step guidance on how to calculate it for various algorithms. We will explain the significance of time complexity, introduce you to Big O notation, discuss asymptotic analysis, and demonstrate how to determine the best and worst-case scenarios.
Additionally, we will examine the relationship between time complexity and space complexity and discuss strategies for optimizing the time complexity of your code. By the end of this guide, you will have a solid understanding of how to calculate and analyze the time complexity of different algorithms.
Key Takeaways:
- Calculating the time complexity of an algorithm is crucial for optimizing the efficiency of your code.
- Time complexity is a measure of the time an algorithm takes to run as a function of the input size.
- Big O notation is used to represent the upper bound of an algorithm’s running time.
- Asymptotic analysis helps analyze algorithm behavior for large input sizes.
- Understanding best and worst-case scenarios is essential for accurate time complexity calculation.
What is Time Complexity?
Before we delve into the intricacies of calculating time complexity, let’s start by understanding what it actually means. In simple terms, time complexity refers to the measure of the amount of time an algorithm takes to execute based on the size of the input it receives. By analyzing the time complexity, we can gain insights into the efficiency of an algorithm and make comparisons between different algorithms to determine their performance.
Big O Notation Explained
When it comes to analyzing the time complexity of an algorithm, Big O notation plays a vital role. It is a widely used notation that helps express the efficiency of an algorithm by representing its upper bound on the running time.
So, what exactly is Big O notation?
Big O notation is a mathematical notation that describes the growth rate of an algorithm’s time complexity as the input size increases. It provides a clear and concise way to analyze and compare different algorithms based on their performance.
In Big O notation, the time complexity of an algorithm is represented by a function, denoted as O(f(n)), where f(n) describes the relationship between the input size (n) and the algorithm’s running time. The ‘O’ in Big O stands for “order,” and it signifies the upper bound or worst-case scenario for the algorithm’s time complexity.
For example, consider an algorithm with a time complexity of O(n^2). This notation indicates that the algorithm’s running time grows quadratically with the input size. In other words, as the input size increases, the running time increases exponentially.
When interpreting Big O notation, it’s important to focus on the highest power of the input size term. This term dominates the growth rate of the function and provides insight into the algorithm’s scalability.
Let’s take a look at some commonly used Big O notations:
Notation | Description |
---|---|
O(1) | Constant time complexity. The algorithm’s running time remains constant regardless of the input size. |
O(log n) | Logarithmic time complexity. The algorithm’s running time increases logarithmically with the input size. |
O(n) | Linear time complexity. The algorithm’s running time increases linearly with the input size. |
O(n^2) | Quadratic time complexity. The algorithm’s running time increases exponentially with the input size. |
O(2^n) | Exponential time complexity. The algorithm’s running time grows exponentially as the input size increases. |
Understanding Big O notation is crucial for analyzing the efficiency of an algorithm and making informed decisions when designing and optimizing code. By identifying the time complexity of an algorithm, developers can anticipate performance bottlenecks, choose the most efficient algorithms, and improve the overall efficiency of their code.
Asymptotic Analysis and its Importance
In the world of algorithm analysis, understanding how an algorithm behaves for large input sizes is crucial. This is where asymptotic analysis comes into play. By examining the scalability of an algorithm and its relationship with input size, asymptotic analysis allows us to determine the time complexity of an algorithm. That’s why it’s so important.
Asymptotic analysis not only helps us evaluate the efficiency and performance of an algorithm but also enables us to compare different algorithms and choose the most suitable one for a specific task.
“The beauty of asymptotic analysis lies in its ability to provide a big picture view of an algorithm’s performance, without getting lost in the nitty-gritty details.”
By focusing on the growth rate of an algorithm as the input size increases, asymptotic analysis helps us identify dominant operations and determine the upper bound on the algorithm’s time complexity. This analysis provides a clear understanding of an algorithm’s behavior and allows us to make informed decisions about its suitability for real-world applications.
Let’s take a closer look at why asymptotic analysis is essential in calculating time complexity:
- Efficiency Evaluation: Asymptotic analysis allows us to objectively evaluate an algorithm’s efficiency, considering the trade-off between time and space requirements. By quantifying the growth rate of an algorithm, we can identify potential performance bottlenecks and optimize them.
- Algorithm Selection: With asymptotic analysis, we can compare different algorithms and select the most efficient one for a specific task. By considering the time complexity of various algorithms, we can choose the one that minimizes execution time and maximizes computational resources.
- Scaling Analysis: Understanding how an algorithm scales with input size is vital for handling large and complex datasets. Asymptotic analysis enables us to predict the performance of an algorithm for massive inputs, helping us design robust and scalable solutions.
Time Complexity Table
Let’s take a look at a table that highlights the most common time complexities encountered in algorithm analysis:
Time Complexity | Description | Examples |
---|---|---|
Constant Time (O(1)) | Algorithm takes the same amount of time regardless of input size. | Accessing an element in an array by index. |
Logarithmic Time (O(log n)) | Algorithm’s running time grows logarithmically with input size. | Binary search on a sorted array. |
Linear Time (O(n)) | Algorithm’s running time increases linearly with input size. | Iterating through an array to find a specific value. |
Quadratic Time (O(n^2)) | Algorithm’s running time increases quadratically with input size. | Performing a nested loop over an array. |
Exponential Time (O(2^n)) | Algorithm’s running time grows exponentially with input size. | Generating all possible subsets of a set. |
Understanding these common time complexities is essential for analyzing and optimizing algorithms to ensure efficient code execution.
By incorporating asymptotic analysis into our algorithm design and analysis process, we can make informed decisions that lead to optimized and effective code.
Basic Steps to Calculate Time Complexity
Now that we have covered the fundamentals of time complexity, let’s explore the practical steps involved in calculating it. By following these simple steps, you can analyze the efficiency of your algorithm and make informed decisions for optimizing its performance.
- Analyze the Code: Begin by carefully examining the code of your algorithm. Identify the parts where most of the computational work is taking place and determine the number of iterations or recursive calls.
- Count the Operations: Next, count the number of operations performed in the code. This could include arithmetic operations, control flow statements, array or list accesses, and function calls.
- Identify the Dominant Term: Look for the term with the highest growth rate in terms of input size. This term will determine the overall time complexity of your algorithm.
- Express the Time Complexity: Use Big O notation to express the time complexity of your algorithm based on the dominant term. Eliminate any constants and lower-order terms, as they become less significant for large input sizes.
Let’s illustrate these steps with an example:
“Consider the following code snippet that calculates the factorial of a number ‘n’:
int factorial(int n) { int fact = 1; for (int i = 1; i
To calculate the time complexity of this code:
- Analyze the Code: The computational work is happening inside the ‘for’ loop where ‘fact’ is multiplied by ‘i’.
- Count the Operations: In each iteration of the loop, a multiplication operation is performed.
- Identify the Dominant Term: The loop runs ‘n’ times, so the dominant term is ‘n’.
- Express the Time Complexity: Using Big O notation, we can express the time complexity as O(n), indicating a linear growth rate with the input size ‘n’.”
By following these steps, you can calculate the time complexity of your algorithms and gain insights into their efficiency. This understanding will help you make informed decisions when it comes to optimizing your code and improving its performance.
Best and Worst Case Scenarios
The time complexity of an algorithm can vary depending on the input data it processes. By considering the best and worst case scenarios, we gain valuable insights into how an algorithm performs in different conditions. Understanding these scenarios is crucial for accurately calculating the time complexity and optimizing algorithm efficiency.
Best Case Scenario
In the best case scenario, the algorithm performs optimally, completing its task in the shortest possible time. This occurs when the input data is well-structured or consists of the most favorable conditions for the algorithm. It represents the lower bound of the time complexity and provides a benchmark for the algorithm’s best performance.
An example of a best case scenario is a search algorithm that finds the target element in the first position of a sorted list. In this case, the algorithm would require only a single comparison to locate the target element, resulting in a time complexity of O(1).
Worst Case Scenario
The worst case scenario represents the upper bound of the time complexity and occurs when the algorithm performs the least efficiently. It happens when the input data is structured in a way that challenges the algorithm’s performance. Understanding the worst case scenario is crucial for ensuring that the algorithm can handle all possible inputs and doesn’t become too slow for critical use cases.
Consider a sorting algorithm that needs to arrange a list of numbers in ascending order. The worst case scenario would be when the list is in reverse order, requiring the algorithm to perform the maximum number of comparisons and swaps. In this case, the time complexity would be O(n^2) for a simple comparison-based sorting algorithm like Bubble Sort.
By analyzing both the best and worst case scenarios, we gain a comprehensive understanding of an algorithm’s time complexity and its performance characteristics under different conditions. This knowledge helps us make informed decisions when selecting or designing algorithms for specific tasks, ensuring optimal efficiency and responsiveness in real-world scenarios.
Space Complexity and Its Relationship to Time Complexity
In addition to time complexity, algorithms also have space complexity, which measures the amount of memory an algorithm requires as a function of the input size. While time complexity focuses on the efficiency of an algorithm in terms of its execution time, space complexity helps us understand the memory usage of the algorithm.
The relationship between space complexity and time complexity is often intertwined. In some cases, optimizing for lower time complexity may result in higher space complexity, and vice versa. This trade-off is known as the time-space complexity trade-off.
Let’s consider an example to illustrate this relationship. The following table shows the time and space complexity of different sorting algorithms:
Sorting Algorithm | Time Complexity | Space Complexity |
---|---|---|
Bubble Sort | O(n^2) | O(1) |
Quick Sort | O(n log n) | O(log n) |
Merge Sort | O(n log n) | O(n) |
In the table above, Bubble Sort has a time complexity of O(n^2) but requires constant space (O(1)). On the other hand, Quick Sort and Merge Sort have better time complexity (O(n log n)) but need additional space for recursion and merging (O(log n) and O(n) respectively).
It’s important to note that the space complexity of an algorithm is not always linearly related to its time complexity. An algorithm with lower time complexity might not necessarily have lower space complexity.
Space Complexity Analysis
When analyzing the space complexity of an algorithm, we consider the memory required for variables, data structures, recursion stacks, and any additional memory usage during the algorithm’s execution.
For example, if an algorithm creates an array of size n and performs operations on it, the space complexity would be O(n) because memory is allocated to store the array.
Similarly, if an algorithm uses a recursive function that makes multiple recursive calls, the space complexity would depend on the maximum depth of recursion, which determines the size of the recursion stack.
Analyze each component of the algorithm and sum up the space required by each component to determine the overall space complexity.
By understanding the relationship between space complexity and time complexity, you can make informed decisions when designing algorithms and optimizing their performance. Balancing the trade-off between time and space complexity allows you to achieve the best possible efficiency and resource usage in your code.
Common Time Complexities and Their Analysis
In the world of algorithms, understanding the time complexity is essential for optimizing code performance. Different algorithms have different time complexities, which directly impact how efficiently they solve problems. In this section, we will explore the most common time complexities and analyze their implications on algorithm performance.
Constant Time Complexity (O(1))
A constant time complexity means that the algorithm takes a constant amount of time to complete, regardless of the input size. This is the most efficient time complexity, as the execution time remains constant, making it highly scalable. An example of an algorithm with constant time complexity is accessing an element from an array by its index.
Linear Time Complexity (O(n))
Linear time complexity means that the execution time of the algorithm grows linearly with the input size. If the input size doubles, the execution time also doubles. This time complexity is considered efficient, but it may not scale well for larger input sizes. An example of an algorithm with linear time complexity is iterating through an array and performing a constant time operation on each element.
Logarithmic Time Complexity (O(log n))
Logarithmic time complexity means that the execution time of the algorithm grows logarithmically with the input size. This time complexity often occurs in divide-and-conquer algorithms, where the input size is halved at each step. Logarithmic time complexity is highly efficient, as it scales well even for large input sizes. An example of an algorithm with logarithmic time complexity is binary search on a sorted array.
Quadratic Time Complexity (O(n^2))
Quadratic time complexity means that the execution time of the algorithm grows quadratically with the input size. This time complexity is considered inefficient and should be avoided for large input sizes. An example of an algorithm with quadratic time complexity is a nested loop that compares each element of one list with every element of another list.
Other Time Complexities
There are many other time complexities, such as cubic time complexity (O(n^3)), exponential time complexity (O(2^n)), and factorial time complexity (O(n!)). These time complexities are increasingly inefficient and should be used with caution, especially for larger input sizes.
Understanding the time complexity of an algorithm is crucial for analyzing its efficiency and scalability. By analyzing the time complexity, we can identify areas for optimization and choose the most appropriate algorithm for a given problem. Let’s summarize the common time complexities:
Time Complexity | Description | Example |
---|---|---|
O(1) | Constant time complexity | Accessing an element from an array by index |
O(n) | Linear time complexity | Iterating through an array |
O(log n) | Logarithmic time complexity | Binary search on a sorted array |
O(n^2) | Quadratic time complexity | Comparing elements of two lists using nested loops |
By knowing the time complexity of an algorithm, we can make informed decisions in algorithm design and optimize code for better performance.
Understanding Nested Loops and Recursion
Nested loops and recursion are powerful programming constructs that can greatly impact the time complexity of an algorithm. By understanding their complexities and analyzing their effects, we can accurately calculate the overall time complexity of an algorithm.
Nested Loops
Nested loops occur when one loop is nested within another. They are commonly used to iterate over multi-dimensional arrays or to perform repetitive tasks within a specified range.
“Nested loops can significantly increase the time complexity of an algorithm, especially when the number of iterations grows exponentially with the input size. It is essential to consider the nested loop structure and analyze its impact on the algorithm’s efficiency.”
When analyzing the time complexity of an algorithm with nested loops, we consider the number of iterations performed by each loop. Let’s consider an example:
“`python for i in range(n): // O(n) for j in range(m): // O(m) # Perform some operation “`
In this example, the outer loop iterates ‘n’ times, and the inner loop iterates ‘m’ times. Therefore, the time complexity of the nested loops is O(n * m).
Recursion
Recursion is a technique where a function calls itself to solve a smaller subproblem. It allows algorithms to solve complex problems by breaking them down into simpler, self-similar subproblems.
“Recursion can lead to elegant and concise code, but it can also result in higher time complexity if not implemented correctly. It is crucial to analyze recursion depth and the number of recursive calls to accurately evaluate the algorithm’s efficiency.”
When analyzing the time complexity of a recursive algorithm, we consider two factors: the number of recursive calls and the work done at each call. Let’s consider an example:
“`python def factorial(n): // O(n) if n
In this example, the recursive function ‘factorial’ calls itself ‘n’ times, performing O(1) work at each call. Therefore, the time complexity of this recursive algorithm is O(n).
Nested Loops vs. Recursion
Both nested loops and recursion can have a significant impact on the time complexity of an algorithm. It is essential to understand the intricacies and analyze their complexities to determine the overall time complexity accurately.
Comparing Nested Loops and Recursion
Construct | Time Complexity |
---|---|
Nested Loops | O(n * m) |
Recursion | O(n) |
Strategies for Optimizing Time Complexity
Once we have calculated the time complexity of an algorithm, we can apply various strategies and techniques to optimize it further. These approaches can significantly improve the time complexity of an algorithm and make it more efficient. Let’s explore some of these strategies:
1. Algorithmic Optimization:
One effective strategy is to analyze the algorithm and identify any redundant or unnecessary operations. By streamlining the algorithm’s logic and eliminating unnecessary steps, we can reduce its time complexity and improve overall efficiency.
2. Data Structure Selection:
The choice of data structure can greatly impact time complexity. By selecting an appropriate data structure for the problem at hand, we can optimize the algorithm’s performance. For example, using a hash table instead of an array for frequent lookups can significantly improve efficiency.
3. Memoization:
Memoization is a technique where we store previously computed results to avoid redundant calculations. By caching intermediate results, we can reduce the time complexity of recursive algorithms, especially those with overlapping subproblems.
4. Greedy Algorithms:
Greedy algorithms aim to make locally optimal choices at each step, resulting in a globally optimal solution. Employing greedy algorithms can often lead to efficient solutions with improved time complexity.
5. Divide and Conquer:
The divide and conquer strategy involves breaking a problem into smaller subproblems, solving them independently, and combining their results to obtain the final solution. This approach can lead to significant time complexity reductions, as it allows for efficient processing of large-scale problems.
6. Dynamic Programming:
Dynamic programming is a powerful technique that breaks complex problems into overlapping subproblems and solves them in a bottom-up manner. By storing intermediate results in a table, we avoid redundant computations and achieve optimized time complexity.
By implementing these strategies and techniques, we can greatly improve the time complexity of our algorithms, enhancing their efficiency and overall performance. Next, we will explore real-life examples and case studies to demonstrate the effectiveness of these optimization strategies.
Conclusion
Congratulations on gaining a solid understanding of how to calculate the time complexity of an algorithm! By knowing how to analyze the efficiency of your code, you can now write more efficient algorithms that can handle larger input sizes with better performance.
Remember, it’s crucial to analyze the time complexity of your algorithms and optimize them when necessary. This will unlock the full potential of your code and ensure that it runs as efficiently as possible.
By applying the concepts of time complexity, such as Big O notation and asymptotic analysis, you can make informed decisions on algorithm design and implementation. This will result in more scalable and optimized solutions.
FAQ
How do I calculate the time complexity of an algorithm?
To calculate the time complexity of an algorithm, you need to analyze the number of basic operations it performs as a function of the input size. This can be done by identifying the loops, recursive calls, and other significant operations in the algorithm and determining how their execution scales with the input. By understanding the algorithm’s behavior and analyzing its operations, you can derive the time complexity.
What is time complexity?
Time complexity is a measure of the amount of time an algorithm takes to run as a function of the input size. It helps us analyze the efficiency of an algorithm and compare different algorithms based on their performance. Time complexity is typically expressed using Big O notation.
What is Big O notation?
Big O notation is a widely used way to express the time complexity of an algorithm. It represents the upper bound on the running time of the algorithm. Big O notation provides an asymptotic analysis of the algorithm’s behavior, focusing on how it scales with the input size rather than the precise amount of time it takes.
Why is asymptotic analysis important for time complexity?
Asymptotic analysis is essential for determining the time complexity of an algorithm. It helps us understand how the algorithm scales with the input size, which is crucial for analyzing its efficiency and performance. By conducting asymptotic analysis, we can identify the dominant factors that contribute to the running time of the algorithm and derive its time complexity.
What are the basic steps to calculate time complexity?
The steps to calculate time complexity involve identifying the significant operations in the algorithm, analyzing their execution frequency, and determining how they scale with the input size. The process includes identifying loops, recursive calls, and other major operations, counting the number of times they execute, and expressing their execution frequency as a mathematical function of the input size.
What are the best and worst case scenarios for time complexity?
The best case scenario refers to the minimum amount of time an algorithm takes to run under the most favorable conditions. It occurs when the input data is already in the desired state or when the algorithm encounters an optimized case. On the other hand, the worst case scenario represents the maximum amount of time an algorithm takes to run under the least favorable conditions. It occurs when the input data is in the most complex state or when the algorithm encounters the least optimized case.
What is space complexity and how does it relate to time complexity?
Space complexity refers to the amount of memory an algorithm requires as a function of the input size. It measures the growth rate of memory consumption with respect to the input size. Space complexity and time complexity are related in the sense that both are measures of efficiency, but they focus on different resources. While time complexity analyzes the computational time required, space complexity analyzes the memory required by the algorithm.
What are the common time complexities and their analysis?
There are several common time complexities, including constant time (O(1)), logarithmic time (O(log n)), linear time (O(n)), quadratic time (O(n^2)), and many more. Each complexity represents a different rate of growth of an algorithm’s running time with respect to the input size. Analyzing these time complexities helps us understand the performance characteristics of different algorithms and choose the most efficient one for a specific problem.
How do nested loops and recursion impact time complexity?
Nested loops and recursion can significantly impact the time complexity of an algorithm. Each nested loop or recursion adds an additional layer of iterations or recursive calls, increasing the overall time complexity. The number of iterations or calls within the nested loops or recursion directly affects the overall time taken by the algorithm. Analyzing these constructs helps us understand the overall time complexity of the algorithm.
What are some strategies for optimizing time complexity?
There are various strategies to optimize the time complexity of an algorithm. Some of these strategies include reducing unnecessary operations, optimizing loops and iterations, using efficient data structures, implementing dynamic programming techniques, and applying mathematical optimizations. By analyzing the time complexity of an algorithm and understanding its bottlenecks, we can apply these strategies to improve its efficiency and reduce the time taken.
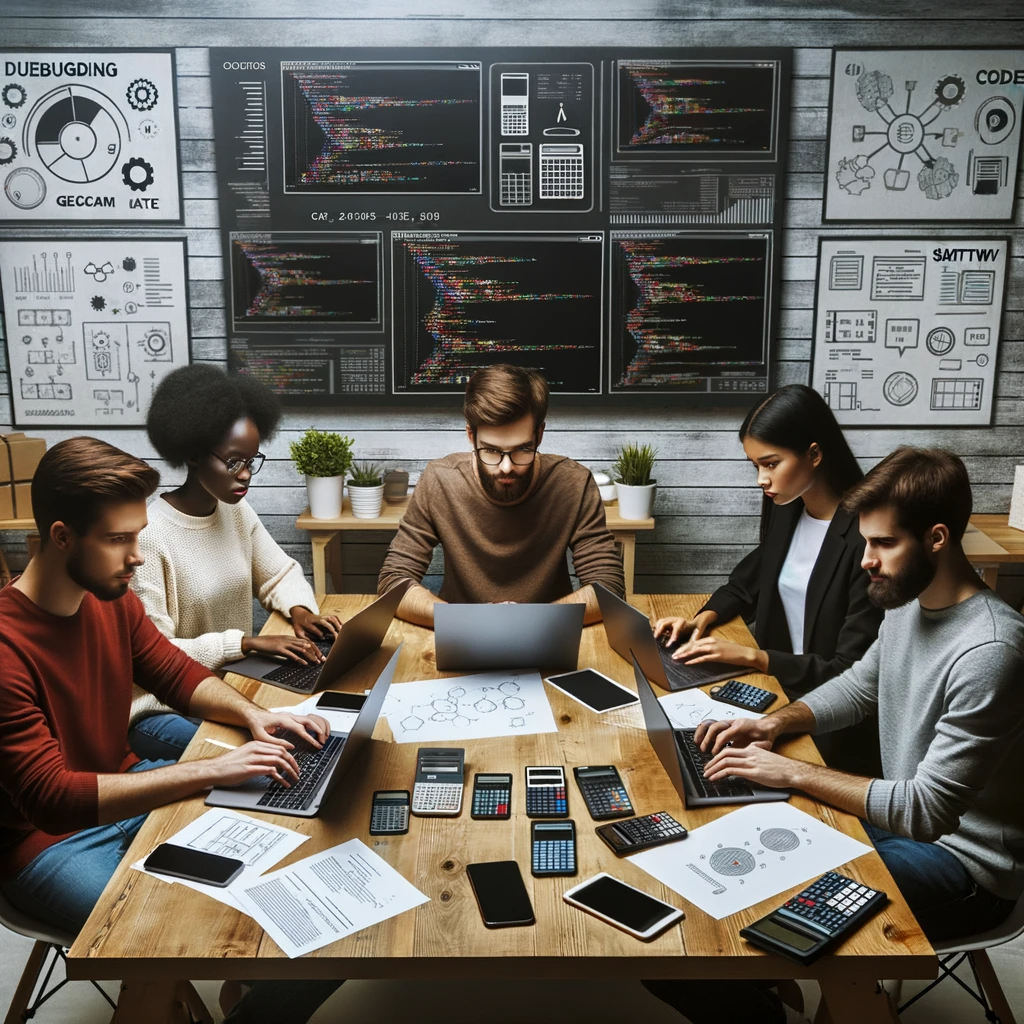
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.