Arrays are fundamental data structures in Java, allowing you to store and manipulate collections of items of the same type. One common operation when working with arrays is to determine their length, i.e., the number of elements they hold. In this blog post, we’ll explore how to calculate the length of an array in Java, along with some best practices and scenarios to keep in mind.
Understanding Array Length:
The length of an array in Java is a fixed value that represents the number of elements within the array. This value is established when the array is created and cannot be changed during the array’s lifetime.
Calculating Array Length:
To calculate the length of an array in Java, you can use the length
property, which is a built-in attribute of every array object. Here’s how you do it:
int[] myArray = {1, 2, 3, 4, 5}; int length = myArray.length; // This gives you the length of the array System.out.println("Array length: " + length);
Best Practices and Tips:
- Use the length Property: Always use the
.length
property to get the length of an array. Avoid calculating it manually using loops, as this property is highly optimized and gives you the correct length directly. - Null Checking: Be cautious when dealing with arrays that might be
null
. Calling.length
on anull
array reference will result in aNullPointerException
, so ensure you’ve properly initialized the array before using it. - Fixed Length: Remember that the length of an array is fixed upon creation. You cannot change it afterward. If you need dynamic sizing, consider using dynamic data structures like
ArrayList
. - Multi-dimensional Arrays: For multi-dimensional arrays (arrays of arrays), the
length
property applies to the outermost array. Inner arrays might have different lengths. Keep this in mind when traversing such arrays. - Array vs. Collection: Java arrays are a low-level construct with fixed sizes. If you need more flexibility and utility, consider using Java Collections like
ArrayList
, which can grow dynamically.
Conclusion:
Calculating the length of an array in Java is a straightforward process using the built-in .length
property. Remember that the length is fixed upon array creation, and using this property provides an efficient and reliable way to retrieve it. By following the best practices outlined in this article, you’ll be better equipped to work with arrays and avoid common pitfalls.
Whether you’re a beginner or an experienced Java programmer, understanding array length calculation is essential for writing efficient and reliable code.
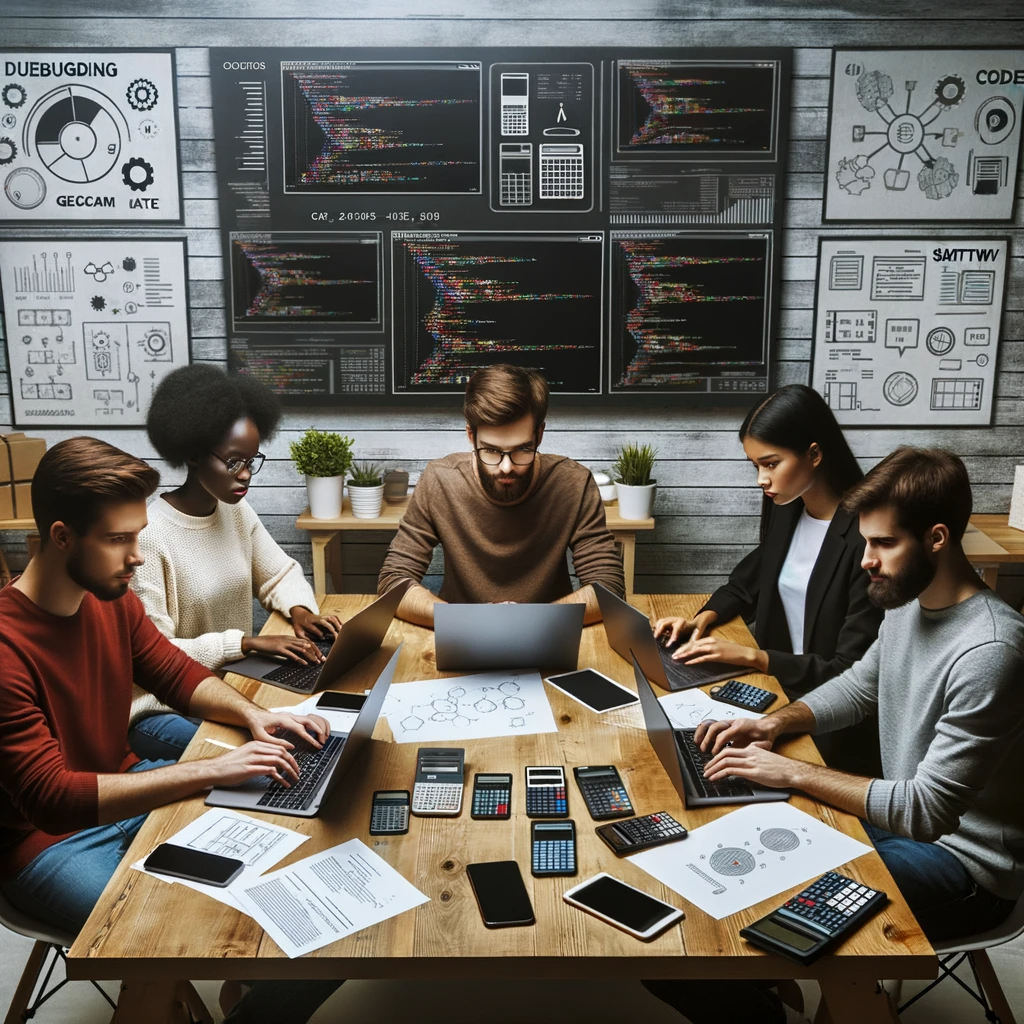
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.