To convert a hexadecimal value to a floating-point number, you can use language-specific functions or methods. In Python, you can use struct.unpack('!f', bytes.fromhex(hex_string))
where hex_string
is the input hexadecimal value. This function converts the hex representation into its IEEE 754 floating-point format and returns the corresponding float value.
Convert Hex to Float Calculator
Result:
Hex Value | Floating-Point Representation | Float Value |
---|---|---|
0x3F800000 | 0 01111111 00000000000000000000 | 1.0 |
0x40490FDB | 0 10000000 10010010000111111011 | 3.14159265 |
0xC0490FDB | 1 10000000 10010010000111111011 | -3.14159265 |
0x7F800000 | 0 11111111 00000000000000000000 | +∞ |
0xFF800000 | 1 11111111 00000000000000000000 | -∞ |
0x7FC00000 | 0 11111111 10000000000000000000 | NaN |
0x00000000 | 0 00000000 00000000000000000000 | +0.0 |
0x80000000 | 1 00000000 00000000000000000000 | -0.0 |
FAQs
How do you convert hex float to decimal? To convert a hexadecimal floating-point number to decimal, you can use the following steps:
- Separate the hexadecimal number into its two parts: the mantissa (fractional part) and the exponent (usually indicated by ‘p’ or ‘e’ followed by an exponent value).
- Convert the mantissa from hexadecimal to decimal.
- Convert the exponent from hexadecimal to decimal.
- Calculate the final decimal value by multiplying the mantissa by 16 raised to the power of the exponent.
How to convert hex to float in C? In C, you can convert a hexadecimal string to a float using the strtof
function. Here’s an example:
cCopy code
#include <stdio.h> #include <stdlib.h> int main() { char hexString[] = "0x3f800000"; // Example hexadecimal string float floatValue = strtof(hexString, NULL); printf("Float value: %f\n", floatValue); return 0; }
How to convert hex string to float in Python? In Python, you can convert a hex string to a float using the struct
module. Here’s an example:
pythonCopy code
import struct hex_string = "0x3f800000" # Example hexadecimal string float_value = struct.unpack('!f', bytes.fromhex(hex_string[2:]))[0] print("Float value:", float_value)
How do you represent a float in hex? A float can be represented in hexadecimal format by converting the binary representation of the float to a hexadecimal string. This can be done using the struct
module in Python or by manually converting the binary components of the float to their hexadecimal equivalents.
How do you convert hex to decimal easily? To convert hex to decimal easily, you can use an online hex to decimal converter or use built-in functions in programming languages like Python, C, or Excel, depending on your needs.
Can Excel convert hex to decimal? Yes, Excel can convert hex to decimal using the HEX2DEC
function. For example, if the hex value is in cell A1, you can use =HEX2DEC(A1)
in another cell to get the decimal equivalent.
How do you convert to float type? In most programming languages, you can convert a value to a float type by explicitly specifying it as a float or by performing a mathematical operation that results in a float. For example, in Python, you can use float()
to convert a value to a float type.
How to convert hex value to decimal in C? To convert a hex value to decimal in C, you can use the strtol
function with a base of 16. Here’s an example:
cCopy code
#include <stdio.h> #include <stdlib.h> int main() { char hexString[] = "1A"; // Example hexadecimal string long decimalValue = strtol(hexString, NULL, 16); printf("Decimal value: %ld\n", decimalValue); return 0; }
How to convert hex to float in Matlab? In MATLAB, you can convert a hexadecimal string to a float using the hex2num
function. Here’s an example:
matlabCopy code
hexString = '3f800000'; % Example hexadecimal string floatValue = hex2num(hexString); disp(['Float value: ' num2str(floatValue)]);
How to convert string variable to float? To convert a string variable to a float, you can use a language-specific function like float()
in Python or strtof()
in C. Here’s a Python example:
pythonCopy code
string_variable = "3.14" # Example string variable float_value = float(string_variable) print("Float value:", float_value)
Can you convert string to float? Yes, you can convert a string to a float in programming languages that support type conversion functions.
How do you convert a hex string to a number? You can convert a hex string to a number (e.g., decimal, binary, or integer) by using language-specific functions or methods to parse and convert the string.
Can hexadecimal be a float? Yes, hexadecimal can represent a floating-point value, often used in IEEE 754 floating-point representation. The hexadecimal format for floats is used to represent the binary structure of a floating-point number.
What is the format code for float? The format code for a float varies depending on the programming language or context. In Python, for example, the format code for a float is 'f'
when using the str.format()
method or in format strings with %
. In C, you might use "%f"
with functions like printf
for formatting floats.
How to convert floating-point hex to binary? To convert a floating-point hexadecimal value to binary, you would first convert the hexadecimal representation to its binary equivalent, considering both the mantissa and the exponent, as per the IEEE 754 standard for floating-point representation.
How to convert hex to decimal in Python? In Python, you can convert a hex string to decimal using the int()
function with a base of 16. Here’s an example:
pythonCopy code
hex_string = "1A" # Example hexadecimal string decimal_value = int(hex_string, 16) print("Decimal value:", decimal_value)
How do you convert hex to integer in Python? To convert hex to an integer in Python, you can use the int()
function with a base of 16, just like in the previous example.
Can hex represent decimals? Yes, hexadecimal can represent decimal numbers, but it is typically used for representing binary data and integers in a more compact and human-readable form.
What is the decimal equivalent of hex? The decimal equivalent of a hexadecimal number depends on the specific hexadecimal value. For example, the decimal equivalent of the hexadecimal number “A” is 10.
What is hex A in decimal? The hexadecimal value “A” is equivalent to the decimal value 10.
Can Excel convert hex to binary? Yes, Excel can convert hex to binary using the DEC2BIN
function. For example, if the hex value is in cell A1, you can use =DEC2BIN(HEX2DEC(A1))
in another cell to get the binary equivalent.
How do you use the float command? The float
command is not standard in most programming languages. Instead, you typically use functions like float()
in Python or strtof()
in C to convert values to float types.
Is float type same as decimal type? No, the float type and decimal type are not the same. The float type represents floating-point numbers using binary representation with limited precision, while the decimal type represents decimal numbers with exact precision. Decimal types are often used for financial calculations to avoid rounding errors.
Is %f used for float? Yes, %f
is a format specifier used in C and some other programming languages for formatting and displaying floating-point numbers with functions like printf
.
How do you convert hex to decimal manually? To convert hex to decimal manually, you can use the following steps:
- Write down the hexadecimal number.
- Assign decimal values to each hex digit from right to left (starting with 0 for the rightmost digit).
- Multiply each digit’s decimal value by 16 raised to the power of its position.
- Sum up all the results from step 3 to get the decimal equivalent.
For example, to convert “1A” to decimal:
- 1A in hex is 116^1 + A16^0 = 16 + 10 = 26 in decimal.
How to convert hex data to ASCII? To convert hex data to ASCII in Python, you can use the binascii
module. Here’s an example:
pythonCopy code
import binascii hex_data = "48656c6c6f2c20576f726c64" # Hex data ascii_data = binascii.unhexlify(hex_data).decode('utf-8') print("ASCII data:", ascii_data)
How to convert hex value to integer in C? To convert a hex value to an integer in C, you can use the strtol
function with a base of 16. Here’s an example, similar to the one mentioned earlier:
cCopy code
#include <stdio.h> #include <stdlib.h> int main() { char hexString[] = "1A"; // Example hexadecimal string int intValue = strtol(hexString, NULL, 16); printf("Integer value: %d\n", intValue); return 0; }
How to convert int RGB to float? To convert integer RGB values (0-255) to float values (0.0-1.0), you can simply divide each integer component by 255.0. For example:
pythonCopy code
red = 128 green = 64 blue = 192 float_red = red / 255.0 float_green = green / 255.0 float_blue = blue / 255.0 print("Float RGB:", float_red, float_green, float_blue)
How to convert from hex to decimal in MATLAB? In MATLAB, you can convert a hex string to decimal using the hex2dec
function. For example:
matlabCopy code
hexString = '1A'; % Example hexadecimal string decimalValue = hex2dec(hexString); disp(['Decimal value: ' num2str(decimalValue)]);
How to convert float to hexadecimal in Java? In Java, you can convert a float to hexadecimal using the Float.floatToRawIntBits()
method to get the binary representation as an integer and then use Integer.toHexString()
to convert it to a hexadecimal string. Here’s an example:
javaCopy code
float floatValue = 3.14f; // Example float value int intValue = Float.floatToRawIntBits(floatValue); String hexString = Integer.toHexString(intValue); System.out.println("Hexadecimal value: 0x" + hexString);
How to convert C string to float? In C, you can convert a string to a float using the atof
function. Here’s an example:
cCopy code
#include <stdio.h> #include <stdlib.h> int main() { char floatString[] = "3.14"; // Example string float floatValue = atof(floatString); printf("Float value: %f\n", floatValue); return 0; }
Which converts string value to float value? The function that converts a string value to a float value varies depending on the programming language. In Python, you can use float()
; in C, you can use atof()
.
Which method is used to convert from string to float? The method used to convert from a string to a float depends on the programming language or library you are using. Common methods include float()
in Python and atof()
in C.
Why can’t I convert a string to a float? You may encounter issues converting a string to a float if the string does not represent a valid numeric value, contains non-numeric characters, or has formatting errors. Additionally, the behavior may vary between programming languages and libraries.
How do you convert a string to a float or double? To convert a string to a float or double in most programming languages, you can use a built-in conversion function. In Python, you can use float()
, and in C/C++, you can use atof()
for floats or strtod()
for doubles.
How to convert array of string to float? To convert an array of strings to an array of floats in Python, you can use a list comprehension or a loop to iterate through the array of strings and apply the float()
conversion function to each string element.
How do you read a hex string? To read a hex string, you can parse it character by character, converting each character to its numeric value. Alternatively, you can use language-specific functions or libraries for hex string manipulation.
How to convert hex string to bytes? In Python, you can convert a hex string to bytes using the bytes.fromhex()
method. Here’s an example:
pythonCopy code
hex_string = "48656c6c6f" # Hex string byte_data = bytes.fromhex(hex_string) print("Byte data:", byte_data)
Does hex return a string? Yes, hexadecimal representation typically returns a string. When you convert a value to its hexadecimal representation, it is represented as a string of hexadecimal digits.
Is hexadecimal 8 bit or 16 bit? Hexadecimal itself does not have a fixed bit width; it’s a numeral system used to represent values in a base-16 format. However, in computing, hexadecimal is often used to represent 8-bit (1 byte) or 16-bit (2 bytes) values, especially in memory addresses and binary data.
Is float 16 or 32 bit? The bit width of a float depends on the floating-point format being used. A standard single-precision float (float) is typically 32 bits, while a double-precision float (double) is typically 64 bits. However, there are other floating-point formats, such as half-precision floats (float16), which are 16 bits.
How many digits can be in a float? The number of digits in a floating-point number (float or double) is not fixed; it depends on the precision of the data type. In single-precision (float), you typically have about 7 decimal digits of precision, while in double-precision (double), you have about 15-16 decimal digits of precision.
How to format float in string? To format a float as a string in various programming languages, you can use functions like str()
or format()
in Python, printf
in C, or libraries like String.format()
in Java.
Is float 32 or 64? A float can refer to either a 32-bit single-precision floating-point number or a 64-bit double-precision floating-point number, depending on the context. Single-precision floats are typically 32 bits, while double-precision floats are typically 64 bits.
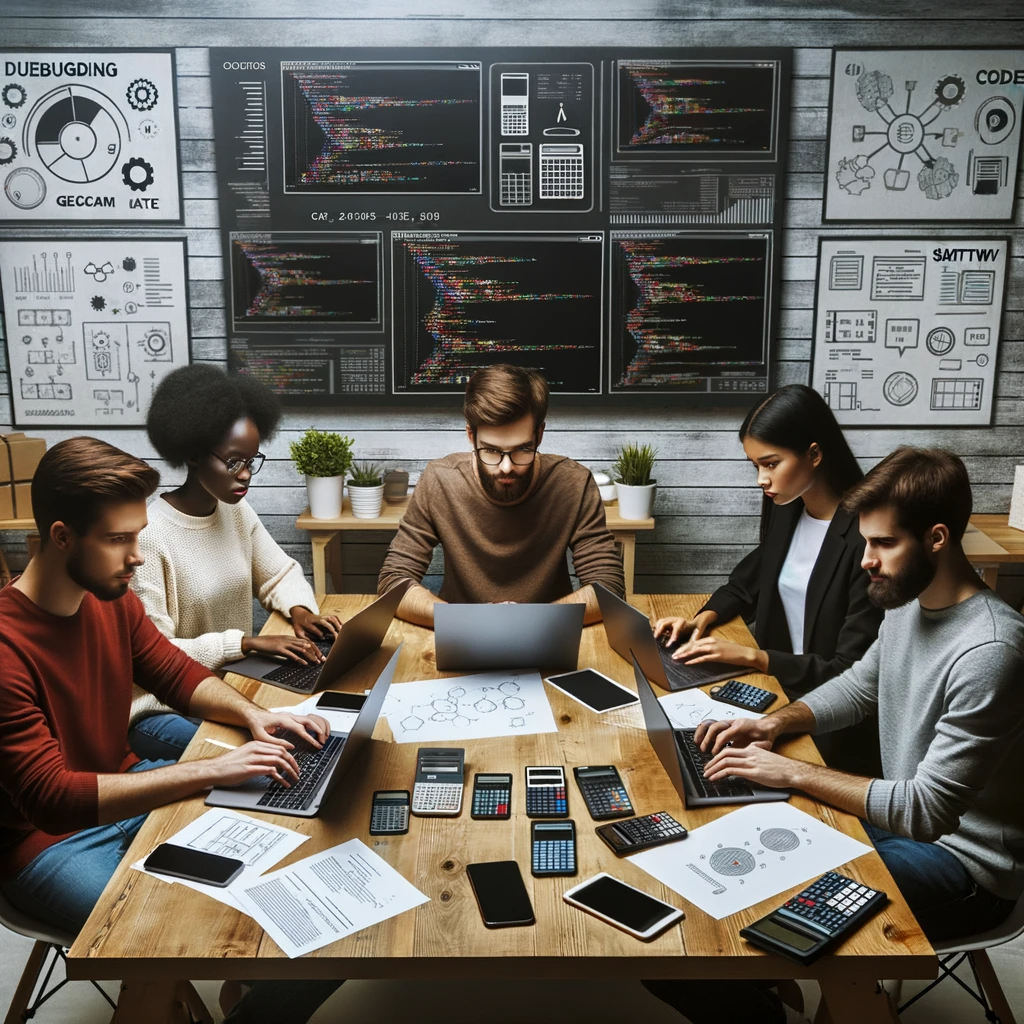
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.