Key Takeaways:
- The height of a binary tree refers to the longest path from the root node to any leaf node.
- Calculating the height of a binary tree is crucial for analyzing and optimizing its performance.
- Two popular approaches to calculate the height of a binary tree are the recursive approach and the iterative approach.
- The recursive approach involves breaking down the problem into subproblems and solving them using recursive calls.
- The iterative approach utilizes algorithms and data structures to efficiently calculate the height of a binary tree.
What is a Binary Tree?
Before we dive into calculating the height of a binary tree, let’s first understand what a binary tree is.
A binary tree is a hierarchical data structure in which each node has at most two children.
It consists of nodes, with each node containing a value and references to its left and right child nodes.
The binary tree follows a specific set of rules, which we will explain further in this section.
Recursive Approach to Calculating Tree Height
One of the most common approaches to calculate the height of a binary tree is using recursion. By utilizing a recursive approach, we can break down the problem into smaller subproblems and solve them iteratively. This recursive solution follows a simple logic that allows us to calculate the height of any binary tree.
The height of a binary tree is determined by the number of edges in the longest path from the root to a leaf node. To calculate the height using recursion, we need to understand the base case and the recursive steps involved.
Base Case:
The base case represents the simplest form of the problem that can be solved directly, without further recursion. In the case of calculating the height of a binary tree, the base case occurs when we reach a leaf node (a node with no children). At this point, the height is 0, as it marks the end of a path.
Recursive Logic:
For non-leaf nodes, the height is determined by the maximum height of its children plus one. We recursively apply this logic to each node in the binary tree until we reach the base case (leaf node). This recursive process allows us to gradually calculate the height of the entire binary tree.
To better understand the recursive approach to calculating tree height, let’s consider an example:
Let’s consider the following binary tree: A / \ B C / \ D E / \ F G
The height of this binary tree is 3. We can calculate it as follows:
- The height of node A is equal to the maximum height of its children (B and C) plus one.
- The height of node B is equal to the maximum height of its children (D and E) plus one.
- The height of node C is equal to 0, as it is a leaf node.
- The height of node D is equal to 0, as it is a leaf node.
- The height of node E is equal to the maximum height of its children (F and G) plus one.
- The height of node F is equal to 0, as it is a leaf node.
- The height of node G is equal to 0, as it is a leaf node.
By applying the recursive logic, we can calculate the height of the entire binary tree as 3.
When implementing the recursive approach in your preferred programming language, it is important to handle the base case and the recursive logic correctly. By understanding the base case and the recursive steps involved, you will be able to calculate the height of any binary tree with ease.
Iterative Approach to Calculating Tree Height
In addition to the recursive approach, an iterative approach can also be used to calculate the height of a binary tree. This approach offers an alternative method that may be more suitable in certain scenarios. Let’s explore the iterative approach in detail.
When applying the iterative approach to calculate the height of a binary tree, we utilize different algorithms and data structures to efficiently traverse the tree and determine its height. Some commonly used techniques include:
- Stack-based approach: In this method, we use a stack to keep track of the nodes as we traverse the tree. We start by pushing the root node onto the stack and iterate until the stack is empty. During each iteration, we process the top node of the stack, pushing its children onto the stack if they exist. By maintaining a variable to track the maximum level reached, we can calculate the height of the tree.
- Queue-based approach: Another iterative approach involves using a queue to perform a level-order traversal of the binary tree. We start by enqueueing the root node and iterate until the queue is empty. During each iteration, we dequeue a node, enqueue its children (if any), and keep track of the current level. By keeping a count of the number of nodes at each level, we can determine the height of the tree.
Both of these iterative approaches provide efficient ways to calculate the height of a binary tree without using recursive function calls. The choice between the stack-based and queue-based approach depends on the specific requirements of your application and the type of binary tree you are working with.
Now, let’s take a look at some code snippets to better understand the implementation of the iterative approach.
Time and Space Complexity of Calculating Tree Height
Understanding the time and space complexity of calculating the height of a binary tree is crucial for evaluating its efficiency. In this section, we will discuss the time and space complexity of both the recursive and iterative approaches. We will analyze the performance implications and provide insights into choosing the most suitable approach based on your specific requirements.
When it comes to calculating the height of a binary tree, the time complexity refers to the amount of time it takes for the algorithm to complete its execution. It is usually measured in terms of the number of operations performed. In the case of the recursive approach, the time complexity can be expressed as O(n), where n represents the number of nodes in the binary tree. This is because, in the worst-case scenario, the algorithm needs to visit all the nodes in the tree to calculate its height.
On the other hand, the iterative approach offers a more efficient time complexity. By using a data structure like a queue or a stack, the algorithm can traverse the tree in a level-by-level fashion, eliminating the need to visit all the nodes in one go. As a result, the time complexity of the iterative approach is O(n), where n is the number of nodes in the tree.
When it comes to space complexity, it refers to the amount of memory required by the algorithm to execute. In the case of the recursive approach, the space complexity is determined by the maximum height of the recursive call stack. If the binary tree is well-balanced, the space complexity of the recursive approach is O(log n), where n is the number of nodes in the tree. However, if the tree is highly unbalanced, the space complexity can be as high as O(n), where n is the number of nodes in the tree.
On the other hand, the iterative approach requires additional memory to store the nodes in the queue or stack. Therefore, the space complexity of the iterative approach is O(n), where n is the number of nodes in the tree.
Considering both the time and space complexities of the recursive and iterative approaches, it is crucial to choose the most suitable approach based on your specific requirements. If memory is a concern and the binary tree is well-balanced, the recursive approach might be a better choice. On the other hand, if time efficiency is crucial, the iterative approach offers a more efficient solution.
Now that we have discussed the time and space complexity of calculating the height of a binary tree using both the recursive and iterative approaches, let’s summarize the key takeaways in the table below:
Approach | Time Complexity | Space Complexity |
---|---|---|
Recursive | O(n) | O(log n) to O(n) |
Iterative | O(n) | O(n) |
Conclusion
In conclusion, calculating the height of a binary tree is a fundamental operation in data structures. It plays a crucial role in analyzing and optimizing the performance of binary trees. Whether you choose the recursive or iterative approach, mastering this skill will greatly enhance your understanding of binary trees and enable you to solve more complex problems.
Throughout this guide, we have provided you with step-by-step instructions and examples to help you accurately calculate the height of any binary tree. By following the recursive or iterative approach, you can easily implement this operation in your programming projects. Not only will it improve your coding skills, but it will also optimize the efficiency of your code.
Now that you have the necessary knowledge and tools, it’s time to put them into practice. Incorporate these techniques into your projects and witness the difference it makes in optimizing your code’s performance. By calculating the height of binary trees effectively, you’ll be able to create more efficient and robust applications that can handle large sets of data.
FAQ
How do you calculate the height of a binary tree?
To calculate the height of a binary tree, you can use either a recursive or iterative approach. Both approaches involve traversing the tree and counting the number of levels or edges from the root to the deepest leaf node.
What is a binary tree?
A binary tree is a hierarchical data structure where each node has at most two children. It consists of nodes, each containing a value and references to its left and right child nodes. These nodes follow specific rules that maintain the hierarchical structure.
What is the recursive approach to calculating tree height?
The recursive approach involves traversing the binary tree using a recursive function. At each node, the function calls itself for the left and right child nodes, keeping track of the maximum height of the subtree. The height is then calculated by finding the maximum between the left and right subtree heights and adding 1.
What is the iterative approach to calculating tree height?
The iterative approach involves using a stack or queue data structure to perform a level-by-level traversal of the binary tree. Starting from the root, you enqueue or push each level of nodes onto the stack or queue. The height is incremented as each level is traversed until all nodes have been visited.
What is the time and space complexity of calculating tree height?
The time complexity of calculating the height of a binary tree using the recursive approach is O(n), where n is the number of nodes in the tree. The space complexity is also O(n) due to the recursive function calls and the function call stack. The iterative approach has a time complexity of O(n) and a space complexity of O(d), where d is the maximum depth of the tree.
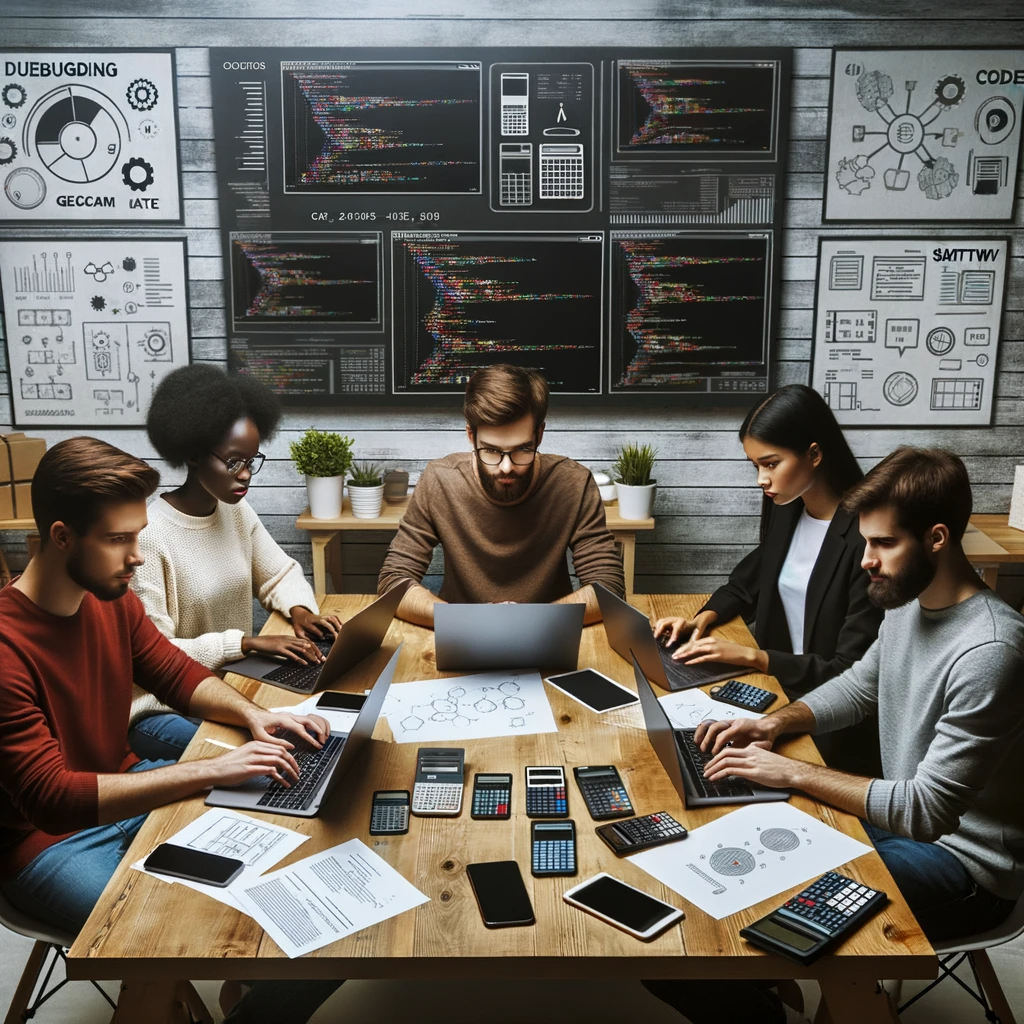
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.