Vector cosine similarity is a similarity metric measuring the cosine of the angle between two vectors. It quantifies their directional similarity, with values from -1 (perfect dissimilarity) to 1 (perfect similarity). Common in text analysis and recommendation systems, it focuses on direction, making it suitable for high-dimensional data like word embeddings.
Vector Cosine Similarity Calculator
Concept | Description |
---|---|
Vector Cosine Similarity | A similarity metric used to measure the cosine of the angle between two vectors. |
Purpose | Measures how similar or dissimilar two vectors are in terms of their direction. |
Range | Values range from -1 (perfect dissimilarity) to 1 (perfect similarity), with 0 indicating no similarity. |
Calculation Formula | Cosine Similarity (cosθ) = (A · B) / ( |
Use Cases | Commonly used in text analysis, information retrieval, recommendation systems, and document similarity. |
Magnitude Insensitivity | Ignores the magnitude (length) of vectors, focusing solely on their direction. |
Parallel Vectors | Cosine similarity between parallel vectors is 1, indicating perfect similarity. |
Perpendicular Vectors | Cosine similarity between perpendicular vectors is 0, indicating perfect dissimilarity. |
Directional Measure | Suitable for comparing document or word embeddings where direction matters more than magnitude. |
Python Calculation Example | python import numpy as np A = np.array([1, 2, 3]) B = np.array([4, 5, 6]) cosine_similarity = np.dot(A, B) / (np.linalg.norm(A) * np.linalg.norm(B)) |
Normalization | Often applied to normalize vectors before cosine similarity calculation. |
Limitations | Doesn't account for magnitude differences, assumes vectors are centered at the origin. |
Comparison to Euclidean Distance | Contrasts with Euclidean distance, which considers both direction and magnitude. |
Applications | Used in various machine learning tasks to measure similarity, e.g., in text clustering and recommendation systems. |
Scalability | Effective in high-dimensional spaces, such as word embeddings. |
FAQs
How do you find the cosine similarity of a vector? Cosine similarity between two vectors is found by taking the dot product of the vectors and dividing it by the product of their magnitudes (Euclidean norms). The formula is:
Cosine Similarity (cosθ) = (A · B) / (||A|| * ||B||)
What is the cosine similarity between two vectors? Cosine similarity measures the cosine of the angle between two vectors. It quantifies how similar or dissimilar two vectors are in terms of their directions.
What is the cosine similarity of two perpendicular vectors? The cosine similarity of two perpendicular vectors is 0. This is because the cosine of the angle between them is cos(90°) = 0, indicating that they are completely dissimilar in direction.
How to find cosine similarity between two vectors in Python? You can find cosine similarity in Python using libraries like NumPy. Here's a sample code snippet:
pythonCopy code
import numpy as np # Define two vectors A and B A = np.array([1, 2, 3]) B = np.array([4, 5, 6]) # Calculate cosine similarity cosine_similarity = np.dot(A, B) / (np.linalg.norm(A) * np.linalg.norm(B)) print(cosine_similarity)
What is the formula for cosine of a vector? The formula for cosine similarity is used to measure the similarity between two vectors, not the cosine of a single vector.
What is the formula for the cosine rule of a vector? The cosine rule is generally used in geometry to find the length of a side or the measure of an angle in a triangle. It's not directly related to vectors in the context of cosine similarity.
Why use cosine similarity instead of Euclidean distance? Cosine similarity is used when you want to measure the similarity of two vectors in terms of direction, ignoring their magnitude. It's suitable for text and high-dimensional data. Euclidean distance considers both direction and magnitude, which may not be appropriate for all data types.
Is cosine similarity always between 0 and 1? No, cosine similarity is not always between 0 and 1. It can be any real number between -1 and 1, inclusive. A value of 1 indicates perfect similarity, 0 indicates no similarity, and -1 indicates perfect dissimilarity.
What is the problem with cosine similarity? Cosine similarity doesn't consider the magnitude of vectors, which can be a problem when the magnitude is important for the similarity measurement. It also assumes that vectors are centered at the origin, which may not always be the case in practical applications.
How do you find the cosine similarity between two sentences? To find the cosine similarity between two sentences, you first represent each sentence as a numerical vector (e.g., using word embeddings), and then apply the cosine similarity formula as mentioned earlier.
What is the difference between Euclidean distance and cosine similarity? Euclidean distance measures the distance between two points in a multidimensional space, taking both direction and magnitude into account. Cosine similarity measures the cosine of the angle between two vectors, focusing on their direction but ignoring magnitude.
How do you find two vectors perpendicular to each other? Two vectors are perpendicular to each other if their dot product is 0. You can find such vectors by choosing one vector and then finding another vector whose dot product with the first vector equals 0.
What is the formula for cosine similarity in word2vec? Word2Vec doesn't directly use cosine similarity in its training. However, after training, you can calculate cosine similarity between word vectors using the standard cosine similarity formula.
How to use the law of cosines to find the angle between two vectors? The law of cosines is typically used to find the angle between sides of a triangle. To find the angle between two vectors using the law of cosines, you'd need to construct a triangle with those vectors and apply the law accordingly, but it's not a common approach for this purpose.
What is the difference between cosine distance and cosine similarity? Cosine similarity measures the cosine of the angle between vectors, resulting in values between -1 and 1, where higher values indicate greater similarity. Cosine distance, on the other hand, is computed as 1 minus the cosine similarity and measures dissimilarity, with values between 0 and 2.
Why do we use cos in vectors? Cosine is used in vectors to measure the similarity or dissimilarity of the directions of vectors, while ignoring their magnitudes. This is valuable in various applications, including text analysis and recommendation systems.
Does cosine rule apply for vectors? The cosine rule is typically applied in the context of triangles to find side lengths or angles. While it can be used with vectors in specific geometric contexts, it's not a standard method for measuring vector similarity like cosine similarity.
What is the basic formula for the cosine function? The basic formula for the cosine function is:
cos(θ) = adjacent side / hypotenuse
In the context of vectors, this formula is adapted for measuring the cosine of the angle between vectors.
What is cosine normalization of a vector? Cosine normalization refers to normalizing a vector by dividing each component of the vector by its Euclidean norm (magnitude), resulting in a unit vector with a magnitude of 1.
What is the cos and sin of a vector? Vectors don't have cos and sin values themselves. Cosine and sine values are typically associated with angles, not vectors. However, in the context of vector angles, you can calculate the cos and sin of the angle between two vectors using the cosine and sine functions.
What is cosine similarity vs Jaccard distance? Cosine similarity measures the similarity of two sets (vectors) in terms of their direction, commonly used for text data. Jaccard distance, on the other hand, measures dissimilarity between sets based on the size of their intersection and union, often used for binary data or sets.
When should you use cosine similarity? Cosine similarity is useful when you want to measure the similarity between vectors (e.g., text documents, word embeddings) in terms of direction while ignoring magnitude. It's commonly used in information retrieval, recommendation systems, and text analysis.
Why Pearson correlation is better than cosine similarity? Whether Pearson correlation is better than cosine similarity depends on the context and the nature of your data. Pearson correlation takes into account both direction and magnitude, making it suitable for data with varying scales and distributions. Cosine similarity only considers direction and is better for data where magnitude is less relevant.
How accurate is cosine similarity? Cosine similarity is accurate for measuring the similarity of vectors in terms of direction. However, its accuracy depends on the quality of vector representations and the relevance of direction as a similarity metric for the specific application.
What is an acceptable cosine similarity? The threshold for an acceptable cosine similarity score depends on the specific application and the problem you're trying to solve. Generally, a cosine similarity close to 1 indicates high similarity, while a score close to 0 indicates low similarity.
What are the advantages of cosine similarity? Advantages of cosine similarity include its ability to measure similarity in high-dimensional spaces, its insensitivity to vector magnitude, and its effectiveness in text analysis and recommendation systems.
Does GPT use cosine similarity? GPT (Generative Pre-trained Transformer) models may not use cosine similarity directly in their architecture, but cosine similarity can be used as a metric to compare the similarity of word embeddings or document representations produced by GPT models.
Can I use cosine similarity as a loss function? Cosine similarity is not commonly used as a loss function in training neural networks. It's typically used as a similarity metric for evaluating the similarity between vectors or representations.
Why cosine similarity is better than dot product? Cosine similarity and the dot product are related but serve different purposes. Cosine similarity measures the similarity of direction between vectors, while the dot product is a mathematical operation that can be used for various purposes, such as calculating projections and similarity scores. The choice between them depends on the specific use case.
Can cosine similarity be negative? Yes, cosine similarity can be negative, especially when dealing with vectors that point in opposite directions. The range of cosine similarity is from -1 to 1, where -1 indicates perfect dissimilarity, 0 indicates no similarity, and 1 indicates perfect similarity.
What is the formula for cosine similarity and distance? The formula for cosine similarity is:
Cosine Similarity (cosθ) = (A · B) / (||A|| * ||B||)
The formula for cosine distance, which is often used as 1 - cosine similarity, is:
Cosine Distance = 1 - Cosine Similarity
Does Bert use cosine similarity? BERT (Bidirectional Encoder Representations from Transformers) is a language model, and it doesn't inherently use cosine similarity. However, cosine similarity can be applied to BERT embeddings to measure text similarity or perform other tasks.
Is Pearson correlation same as cosine similarity? No, Pearson correlation and cosine similarity are not the same. Pearson correlation measures the linear correlation between two variables, considering both direction and magnitude. Cosine similarity measures the cosine of the angle between vectors, focusing on direction while ignoring magnitude.
Is cosine similarity the same as correlation? No, cosine similarity is not the same as correlation. Cosine similarity measures the similarity of directions between vectors, while correlation (such as Pearson correlation) measures the linear relationship between two variables, considering both direction and magnitude.
Is Euclidean distance better than cosine? Euclidean distance and cosine similarity serve different purposes. Euclidean distance measures the distance between two points in space, considering both direction and magnitude. Cosine similarity measures similarity in terms of direction but ignores magnitude. The choice between them depends on the specific problem and data.
How do you know if vectors are parallel or orthogonal? Two vectors are parallel if their cross product is zero, and they are orthogonal (perpendicular) if their dot product is zero. In other words, if v and w are vectors, v · w = 0 for orthogonality, and v × w = 0 for parallelism.
How do you know if vectors are parallel or perpendicular? Vectors are parallel if their directions are the same (or opposite) and perpendicular if their dot product is 0, indicating they are at right angles to each other.
How do you find a vector perpendicular to both A and B? To find a vector that is perpendicular to both A and B, you can take the cross product of A and B. The result will be a vector that is orthogonal to both A and B.
Why does word2vec use cosine similarity? Word2Vec uses cosine similarity to measure the similarity of word vectors because it focuses on the direction of word representations while disregarding their magnitude. This makes it suitable for capturing semantic relationships between words.
What is an example of a cosine similarity word embedding? In word embeddings, cosine similarity is often used to measure the similarity between word vectors. For example, the cosine similarity between the word vectors for "king" and "queen" might be high because they are similar in meaning, while the similarity between "king" and "car" would be lower.
How do you find the cosine similarity in NLP? In NLP, you can find cosine similarity by representing text documents as numerical vectors (e.g., using word embeddings like Word2Vec or TF-IDF), and then applying the cosine similarity formula to these vectors to measure their similarity.
How do you find the direction of cosines and angles of a vector? To find the direction cosines of a vector, divide each component of the vector by the vector's magnitude (Euclidean norm). To find the angle of a vector, you can use the arccosine function and the direction cosines.
What is the angle between two vectors p → 2i ∧ 3j ∧ k ∧ and → − 3i ∧ 6k ∧? To find the angle between two vectors, you can use the cosine of the angle formula:
cos(θ) = (A · B) / (||A|| * ||B||)
Let A be 2i + 3j + k and B be -3i + 6k. Calculate the dot product and magnitudes, then use the formula to find the angle θ.
How to find the angle between two vectors from the resultant vector? To find the angle between two vectors from the resultant vector, you need the vectors themselves, not just their resultant. You can use the dot product formula to find the cosine of the angle between them.
Can cosine similarity be greater than 1? No, cosine similarity cannot be greater than 1. Its range is between -1 and 1, where values close to 1 indicate high similarity, 0 indicates no similarity, and -1 indicates perfect dissimilarity.
Is there a relationship between Euclidean distance and cosine similarity? There is a relationship in that they both measure aspects of similarity between vectors, but they have different interpretations and purposes. Euclidean distance measures the actual distance between two points in space, while cosine similarity measures similarity in terms of direction.
Is cosine similarity machine learning? Cosine similarity itself is not a machine learning algorithm; it's a mathematical concept used in various machine learning applications to measure the similarity between data points, such as text documents or vectors.
When would you use the COS rule? The law of cosines (COS rule) is used in geometry to find side lengths or angles in triangles when you have information about the lengths of sides and the measures of other angles but not enough information to apply the simpler laws of sines and cosines.
What are the 3 formulas for the Law of Cosines? The law of cosines provides three formulas:
- To find the length of one side of a triangle when you know the lengths of the other two sides and the included angle:c² = a² + b² - 2ab * cos(C)
- To find the length of one side of a triangle when you know the lengths of the other two sides and the angle opposite the side you're finding:a² = b² + c² - 2bc * cos(A)
- To find the length of one side of a triangle when you know the lengths of the other two sides and the angle opposite the side you're finding:b² = a² + c² - 2ac * cos(B)
What is vector cosine? Vector cosine typically refers to the cosine of the angle between two vectors. It quantifies how similar the directions of the vectors are.
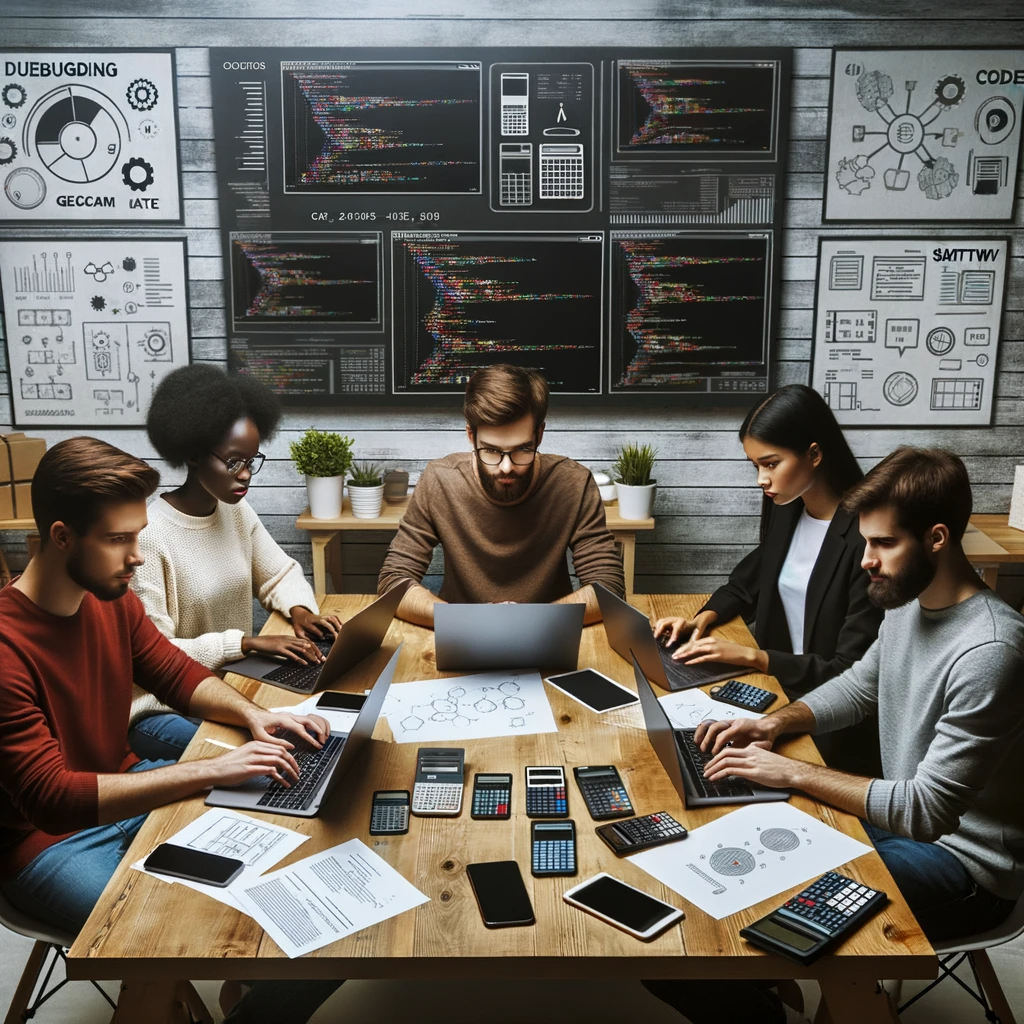
GEG Calculators is a comprehensive online platform that offers a wide range of calculators to cater to various needs. With over 300 calculators covering finance, health, science, mathematics, and more, GEG Calculators provides users with accurate and convenient tools for everyday calculations. The website’s user-friendly interface ensures easy navigation and accessibility, making it suitable for people from all walks of life. Whether it’s financial planning, health assessments, or educational purposes, GEG Calculators has a calculator to suit every requirement. With its reliable and up-to-date calculations, GEG Calculators has become a go-to resource for individuals, professionals, and students seeking quick and precise results for their calculations.